By: CS2113-T16-4
Since: Aug 2018
Licence: MIT
- 1. Setting up
- 2. Design
- 3. Implementation
- 4. Documentation
- 5. Testing
- 6. Dev Ops
- Appendix A: Suggested Programming Tasks to Get Started
- Appendix B: Product Scope
- Appendix C: User Stories
- Appendix D: Use Cases
- Appendix E: Non Functional Requirements
- Appendix F: Glossary
- Appendix G: Instructions for Manual Testing
- G.1. Launch and Shutdown
- G.2. Adding an employee
- G.3. Editing an employee
- G.4. Filtering employees of a certain department and position
- G.5. Finding a specific employee
- G.6. Selecting an employee
- G.7. Modifying an employee’s pay
- G.8. Modifying the pay of all the employees in the list
- G.9. Add Schedule
- G.10. Add Works
- G.11. Add Leaves
- G.12. Calculate Leaves
- G.13. Select Schedule
- G.14. Delete Schedule
- G.15. Delete Works
- G.16. Delete Leaves
- G.17. Add Expenses
- G.18. Delete Expenses
- G.19. Select Expenses
- G.20. Add A Recruitment Post
- G.21. Edit A Recruitment Post
- G.22. Select A Recruitment Post
- G.23. Delete A Recruitment Post
- G.24. Deleting an employee
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
1.3. Verifying the setup
-
Run the
seedu.address.MainApp
and try a few commands -
Run the tests to ensure they all pass.
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
After forking the repo, the documentation will still have the SE-EDU branding and refer to the se-edu/addressbook-level4
repo.
If you plan to develop this fork as a separate product (i.e. instead of contributing to se-edu/addressbook-level4
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
-
Take a look at Appendix A, Suggested Programming Tasks to Get Started.
2. Design
2.1. Architecture
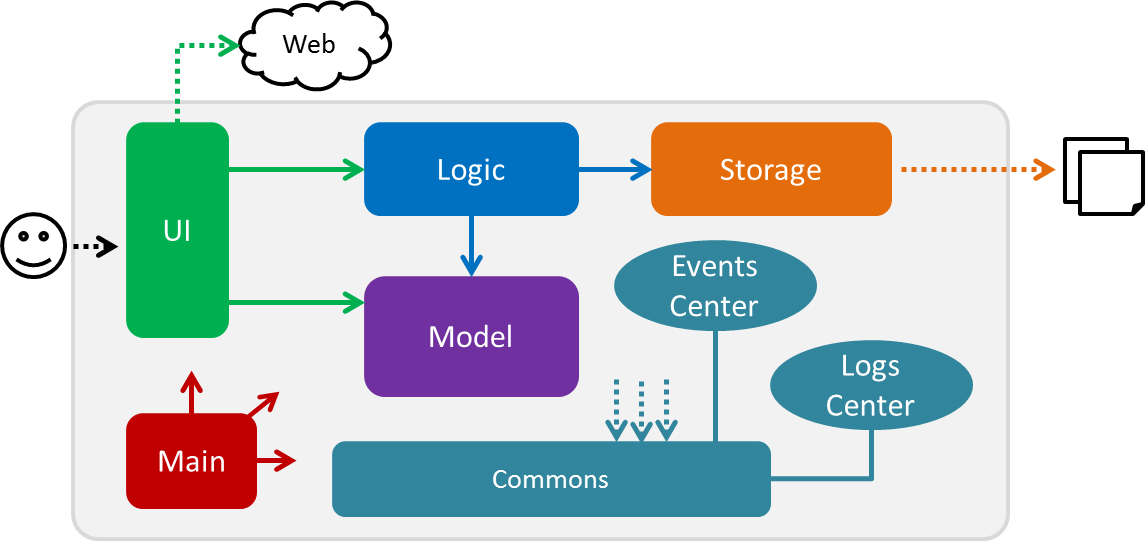
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
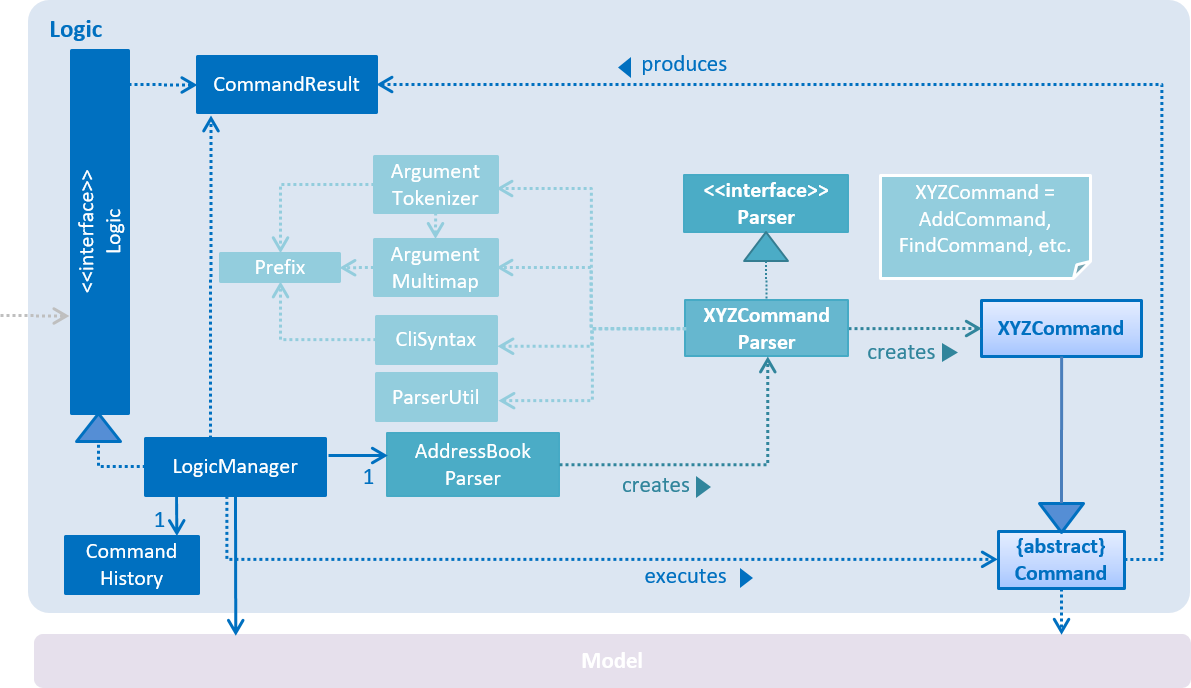
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command delete 1
.
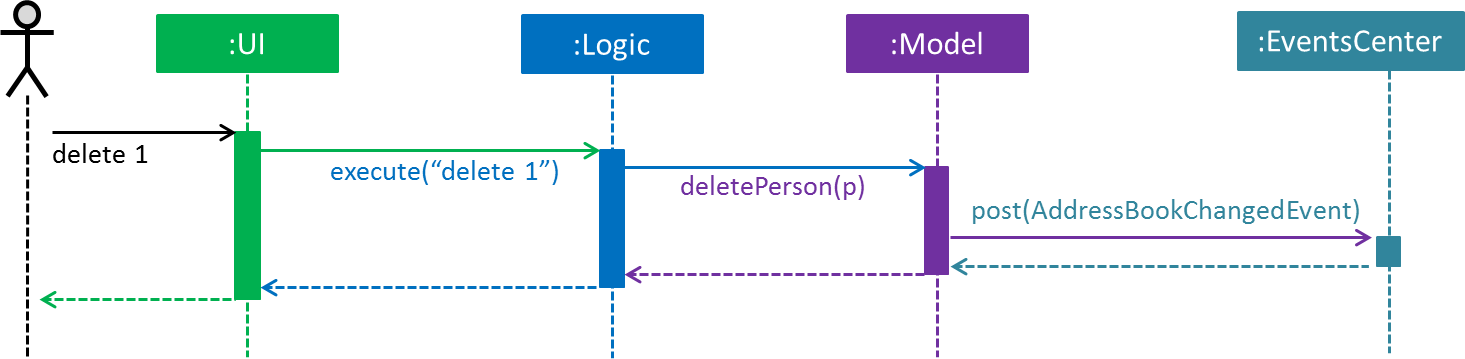
delete 1
command (part 1)
Note how the Model simply raises a AddressBookChangedEvent when the Address Book data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
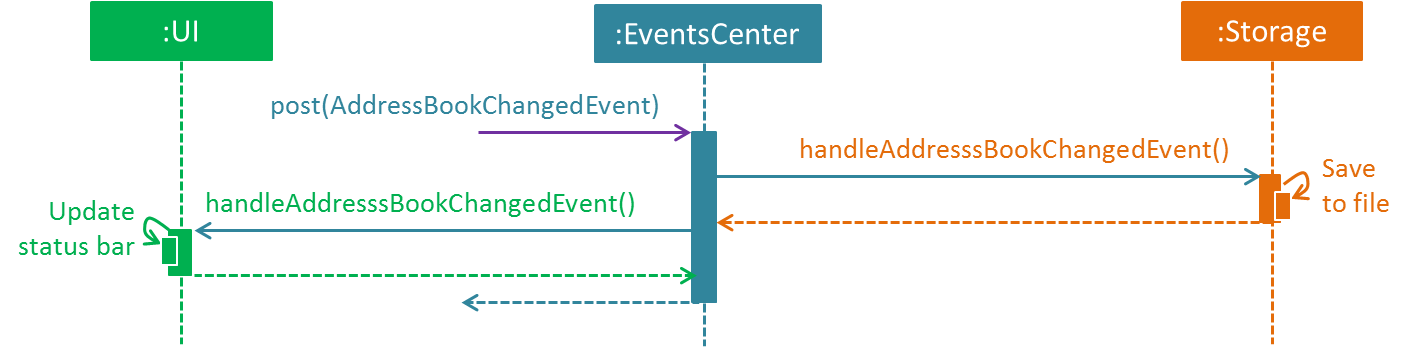
delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
2.2. UI component
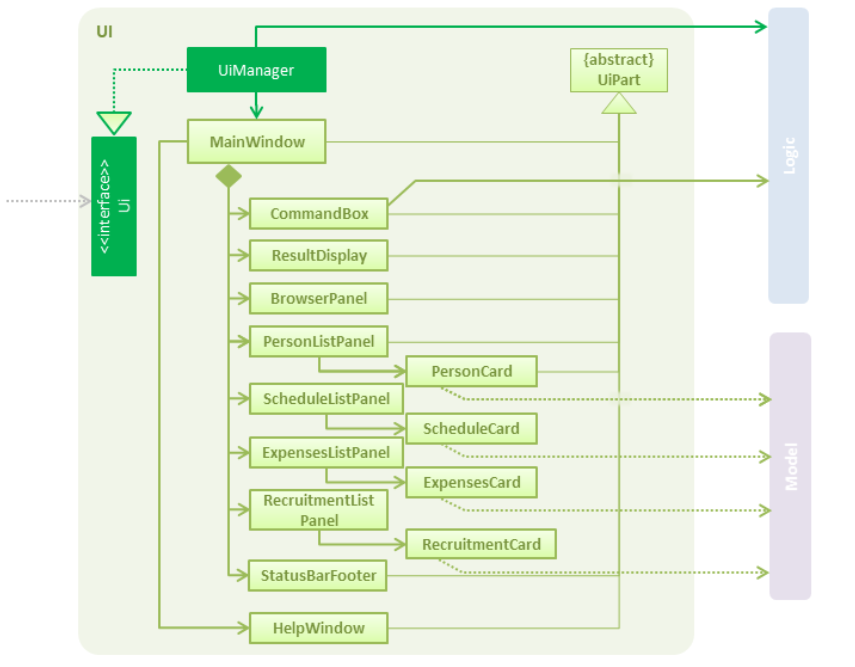
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
, BrowserPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
2.3. Logic component
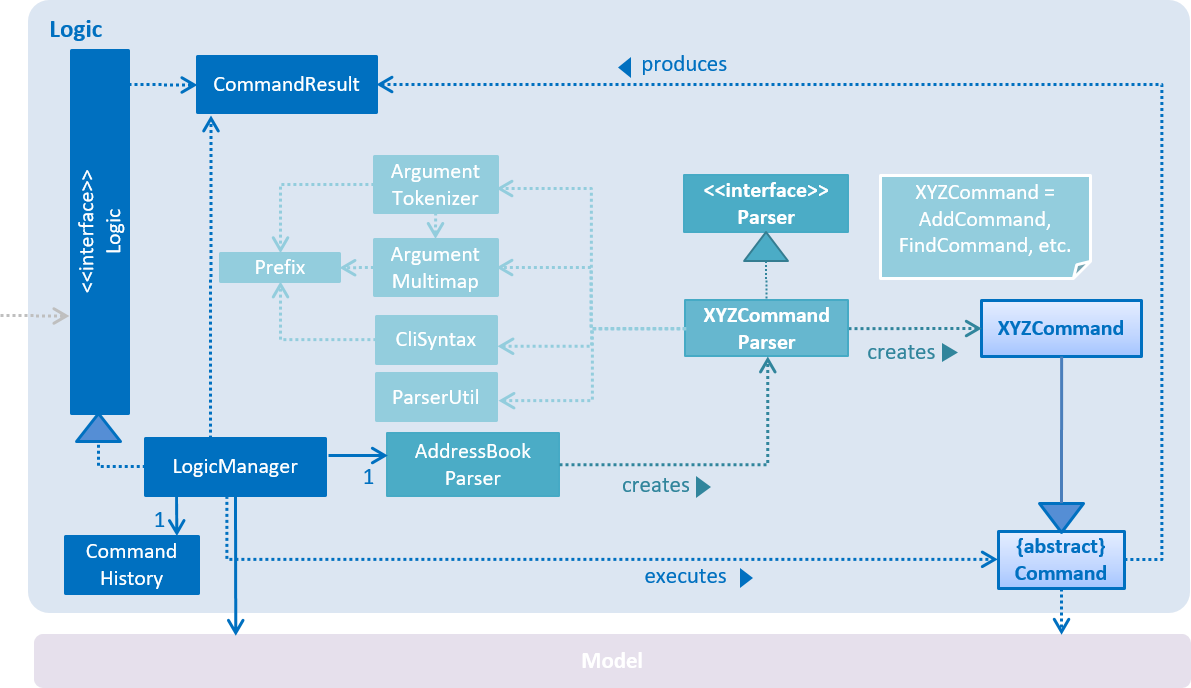
API :
Logic.java
-
Logic
uses theAddressBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a person) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
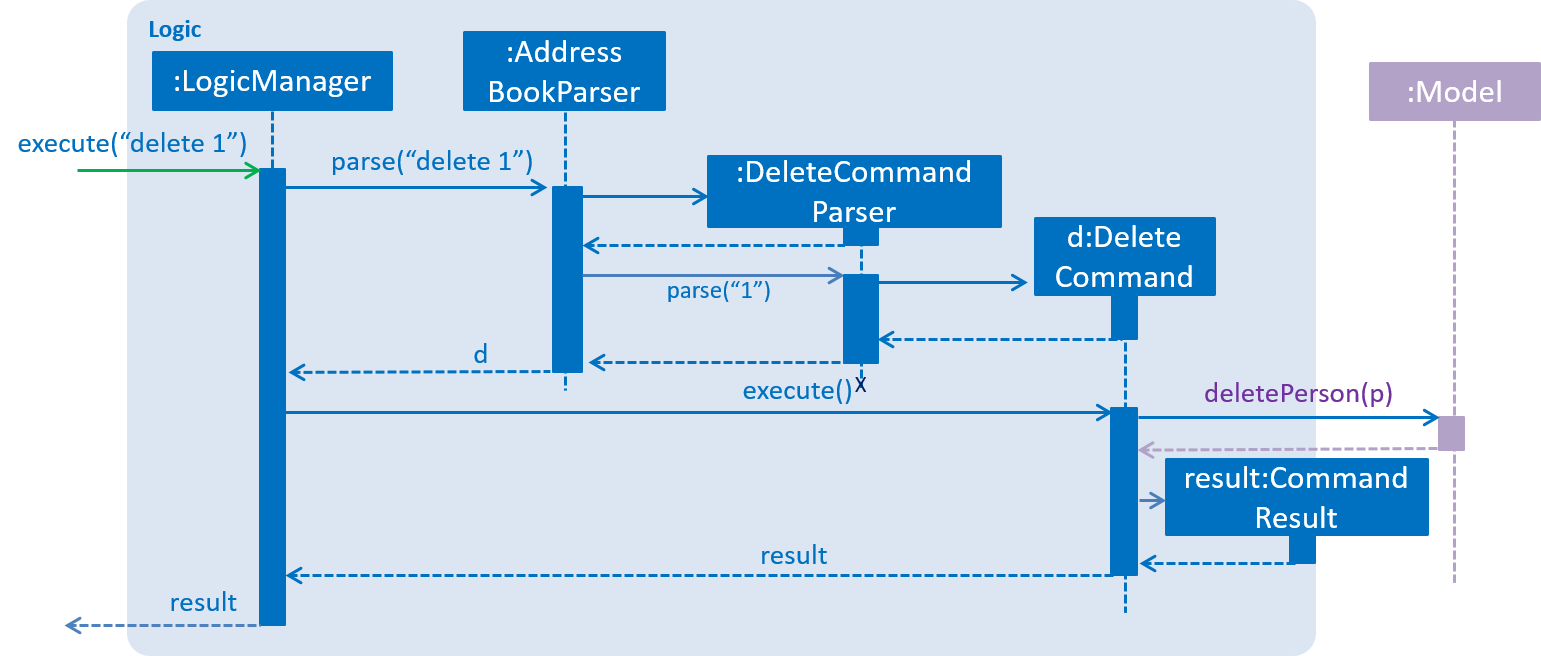
delete 1
Command2.4. Model component
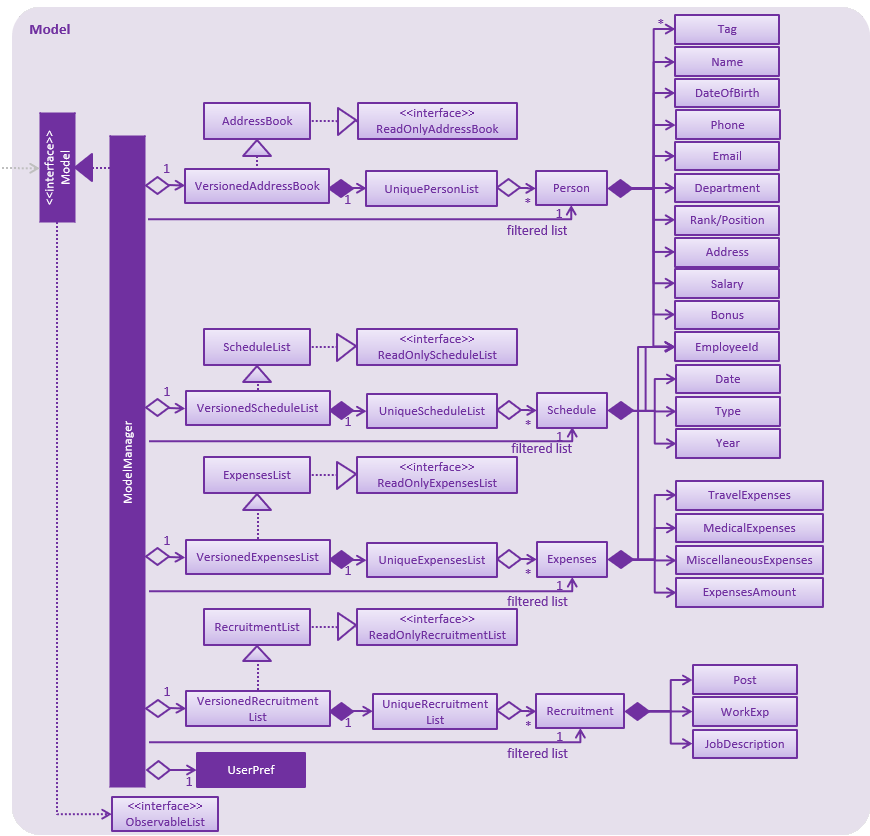
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Address Book, Schedule List, Expenses List and Recruitment List data.
-
exposes unmodifiable
ObservableList<Person>
,ObservableList<Schedule>
,ObservableList<Expenses>
andObservableList<Recruitment>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
As a more OOP model, we can store a Tag list in Address Book , which Person can reference. This would allow Address Book to only require one Tag object per unique Tag , instead of each Person needing their own Tag object. An example of how such a model may look like is given below.![]() |
2.5. Storage component
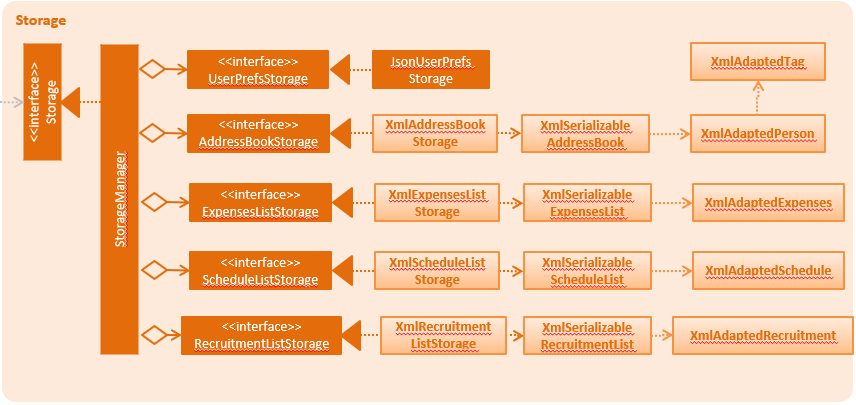
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the Address Book data in xml format and read it back.
-
can save the Expenses List data in xml format and read it back.
-
can save the Schedule List data in xml format and read it back.
-
can save the Recruitment List data in xml format and read it back.
2.6. Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Undo/Redo feature
3.1.1. Current Implementation
The undo/redo mechanism is facilitated by VersionedModelList
, which oversees all the undo and redo for other
storage, namely, VersionedAddressBook
, VersionedScheduleList
, VersionedExpensesList
and VersionedRecruitmentList
.
VersionedModelList
stored internally as an modelTypesStateList
and currentStatePointer
.
Additionally, it implements the following operations:
-
VersionedAddressBook#add()
— Save the storage model type that has committed in its history. -
VersionedAddressBook#addMultiple()
— Saves the set of storage model types that has committed in its history. -
VersionedAddressBook#undo()
— DelegateVersionedAddressBook
,VersionedScheduleList
,VersionedExpensesList
andVersionedRecruitmentList
to restore the previous storage model state from its history. -
VersionedAddressBook#redo()
— DelegateVersionedAddressBook
,VersionedScheduleList
,VersionedExpensesList
andVersionedRecruitmentList
to restore a previously undone address book state from its history.
The following is an example of VersionedAddressBook
implementation. The implementation will
be similar to VersionedScheduleList
, VersionedExpensesList
and VersionedRecruitmentList
.
VersionedAddressBook
extends AddressBook
with an undo/redo history, stored internally as an addressBookStateList
and currentStatePointer
.
Additionally, it implements the following operations:
-
VersionedAddressBook#commit()
— Saves the current address book state in its history. -
VersionedAddressBook#undo()
— Restores the previous address book state from its history. -
VersionedAddressBook#redo()
— Restores a previously undone address book state from its history.
These operations are exposed in the Model
interface as Model#commitAddressBook()
, Model#undoAddressBook()
and Model#redoAddressBook()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedAddressBook
will be initialized with the initial address book state, and the currentStatePointer
pointing to that single address book state.

Step 2. The user executes delete 5
command to delete the 5th person in the address book. The delete
command calls Model#commitAddressBook()
, causing the modified state of the address book after the delete 5
command executes to be saved in the addressBookStateList
, and the currentStatePointer
is shifted to the newly inserted address book state.
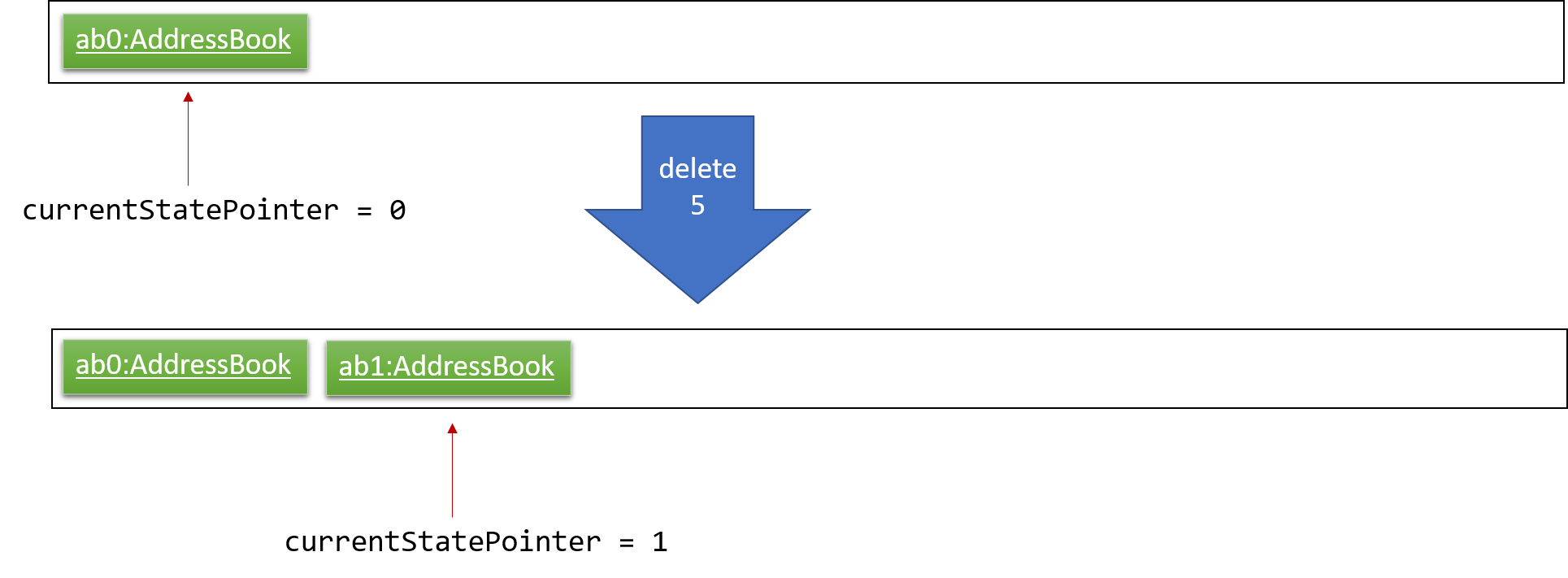
Step 3. The user executes add n/David …
to add a new person. The add
command also calls Model#commitAddressBook()
, causing another modified address book state to be saved into the addressBookStateList
.
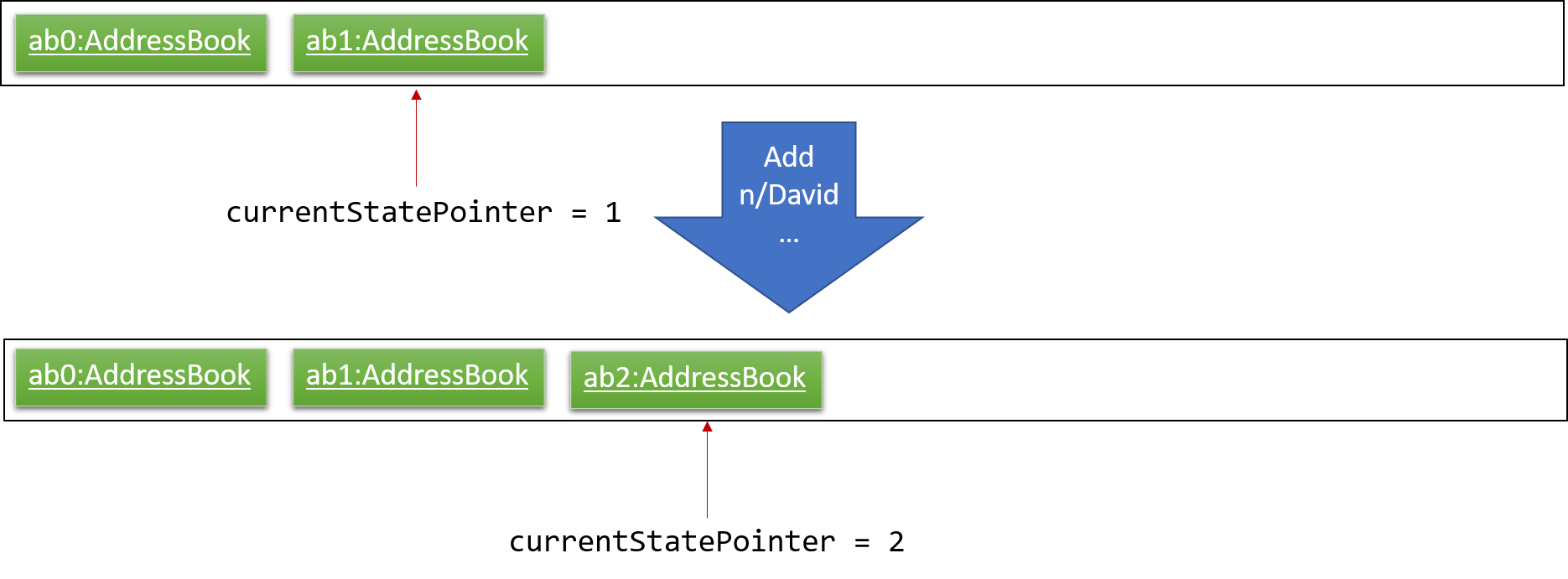
If a command fails its execution, it will not call Model#commitAddressBook() , so the address book state will not be saved into the addressBookStateList .
|
Step 4. The user now decides that adding the person was a mistake, and decides to undo that action by executing the undo
command. The undo
command will call Model#undoAddressBook()
, which will shift the currentStatePointer
once to the left, pointing it to the previous address book state, and restores the address book to that state.
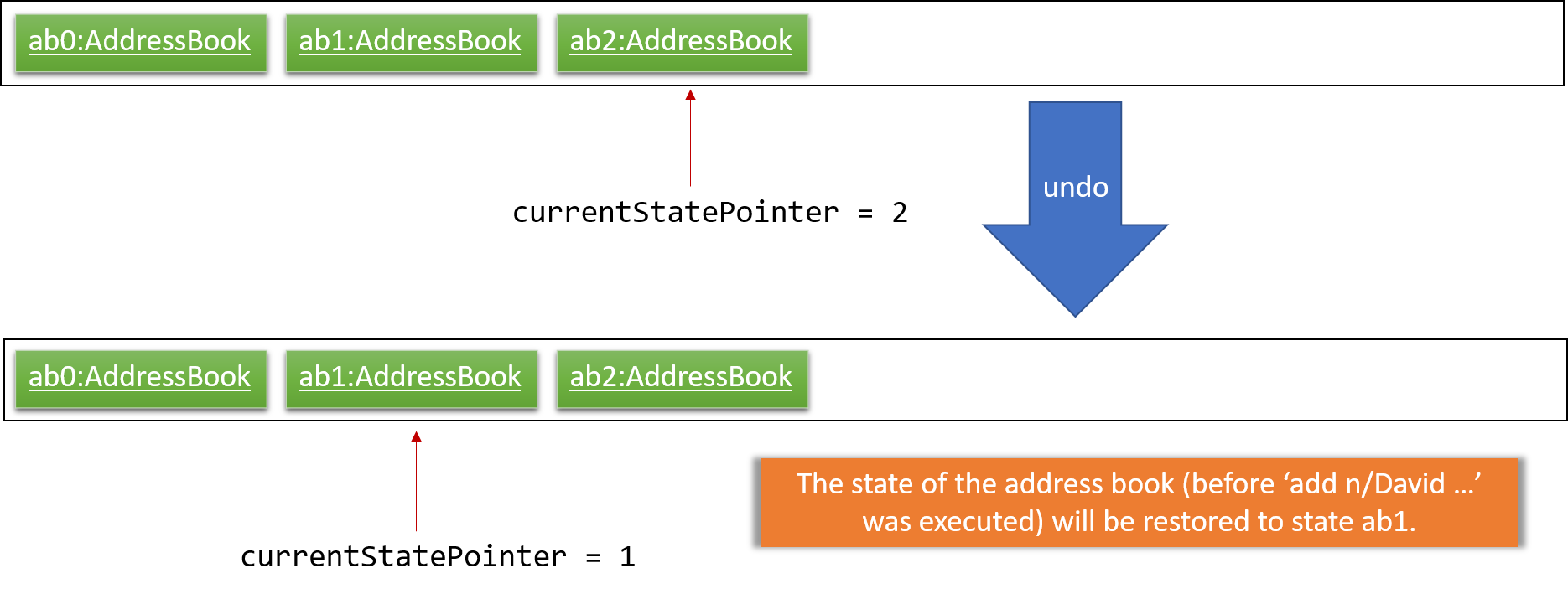
If the currentStatePointer is at index 0, pointing to the initial address book state, then there are no previous address book states to restore. The undo command uses Model#canUndoAddressBook() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the undo.
|
The following sequence diagram shows how the undo operation works:
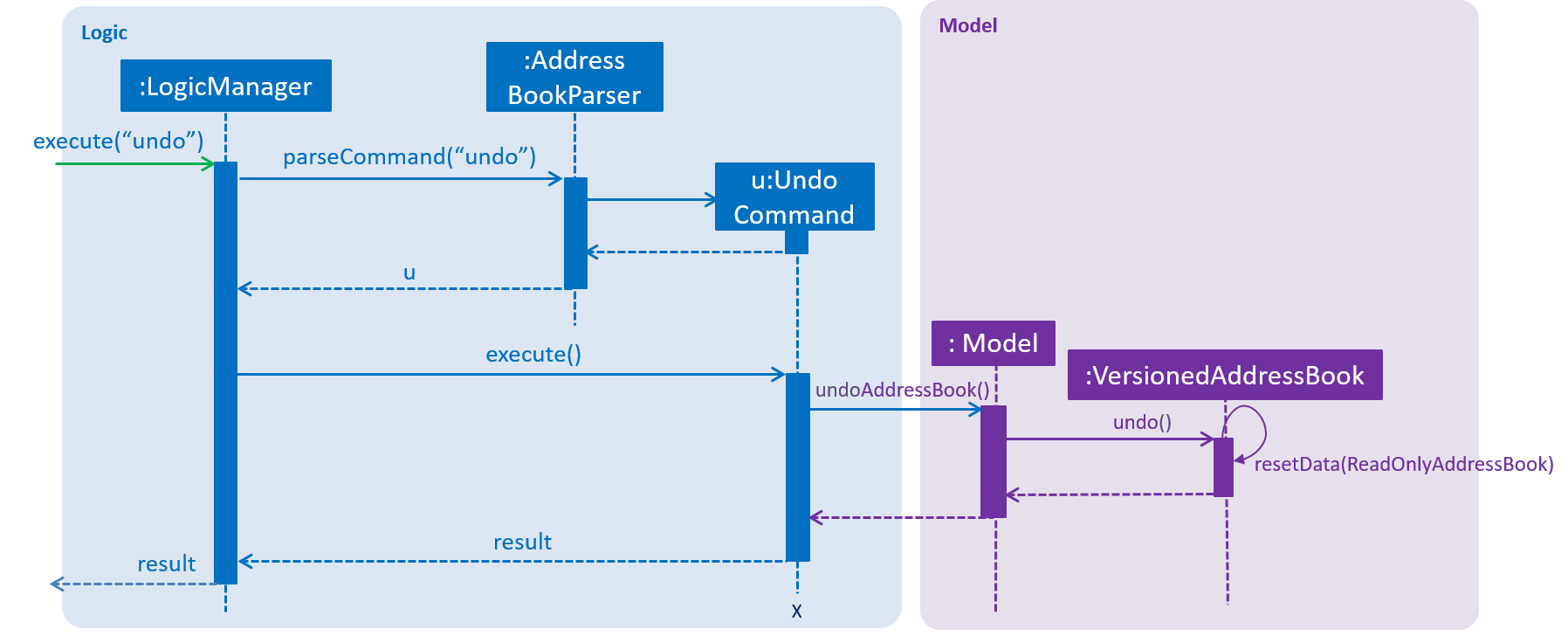
The redo
command does the opposite — it calls Model#redoAddressBook()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores the address book to that state.
If the currentStatePointer is at index addressBookStateList.size() - 1 , pointing to the latest address book state, then there are no undone address book states to restore. The redo command uses Model#canRedoAddressBook() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
|
Step 5. The user then decides to execute the command list
. Commands that do not modify the address book, such as list
, will usually not call Model#commitAddressBook()
, Model#undoAddressBook()
or Model#redoAddressBook()
. Thus, the addressBookStateList
remains unchanged.
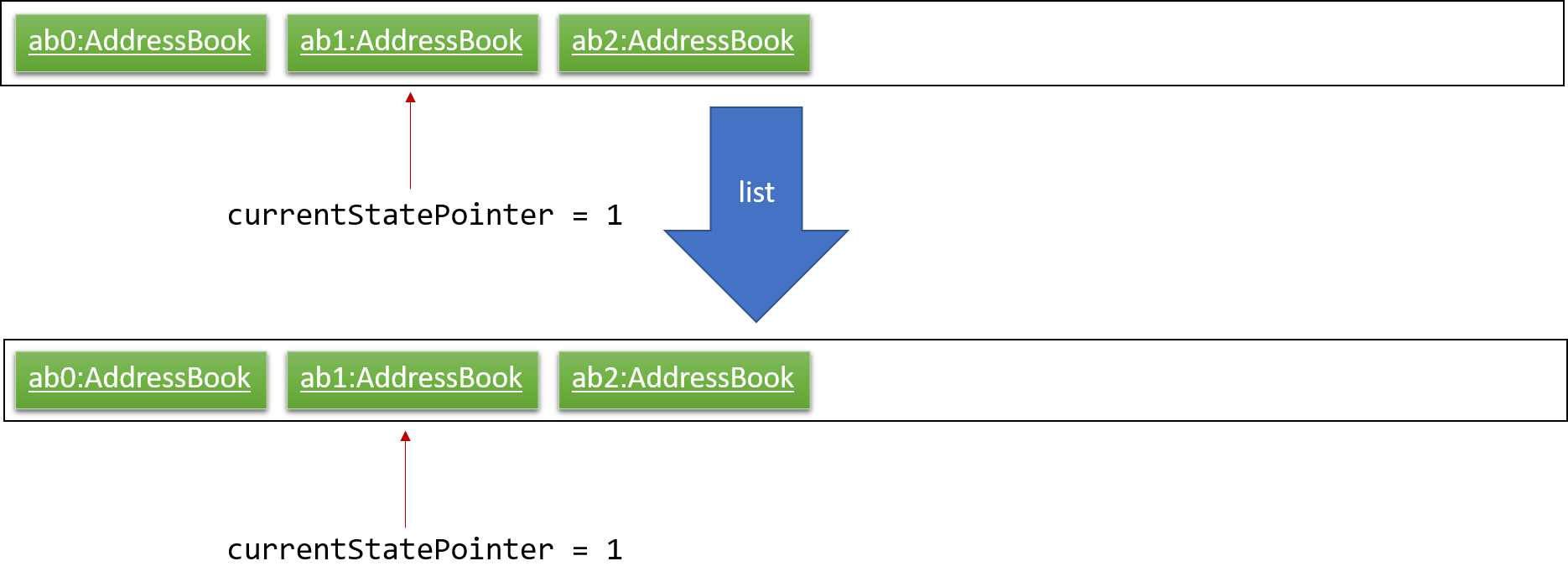
Step 6. The user executes clear
, which calls Model#commitAddressBook()
. Since the currentStatePointer
is not pointing at the end of the addressBookStateList
, all address book states after the currentStatePointer
will be purged. We designed it this way because it no longer makes sense to redo the add n/David …
command. This is the behavior that most modern desktop applications follow.
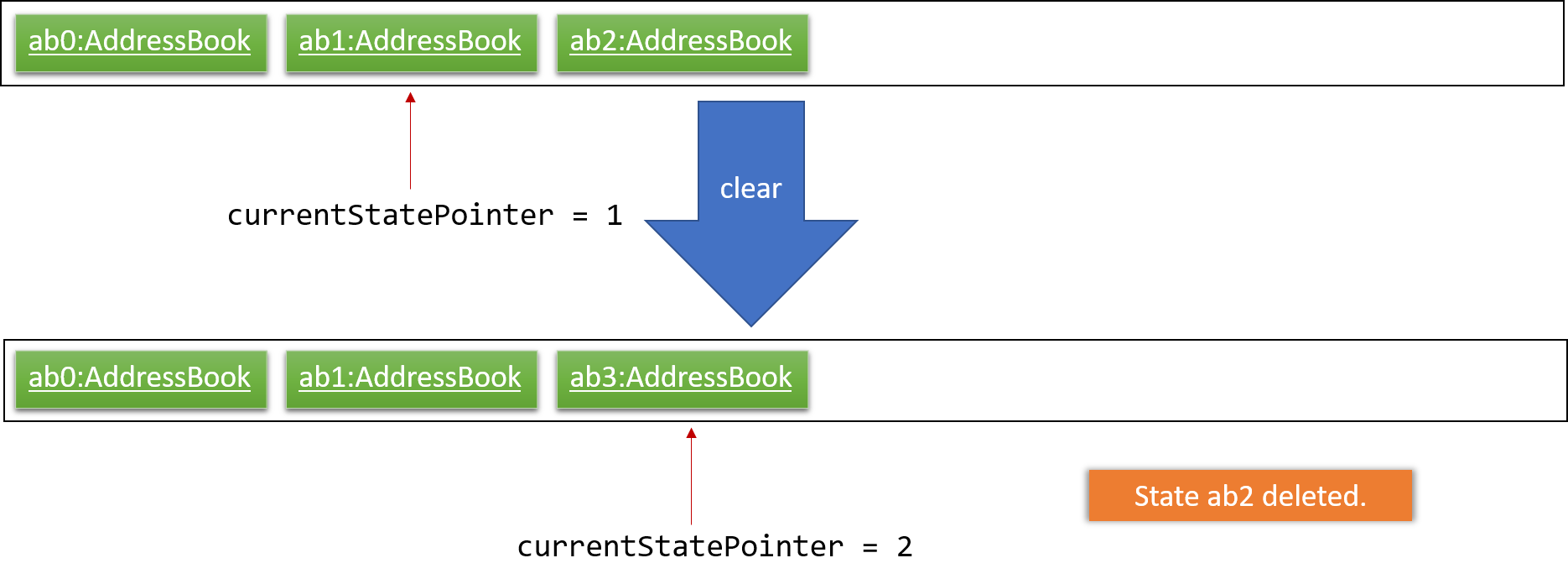
The following activity diagram summarizes what happens when a user executes a new command:
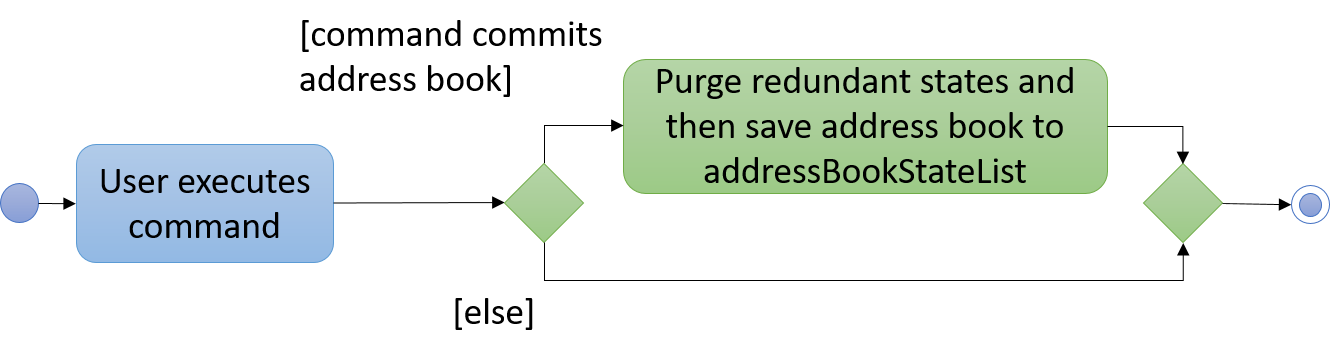
3.1.2. Design Considerations
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire address book.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
delete
, just save the person being deleted). -
Cons: We must ensure that the implementation of each individual command are correct.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use a list to store the history of address book states.
-
Pros: Easy for new Computer Science student undergraduates to understand, who are likely to be the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update both
HistoryManager
andVersionedAddressBook
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate list, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as
HistoryManager
now needs to do two different things.
-
3.2. Modify Pay Feature
The command modifyPay
allows the user to modify the salary and bonus of the employee based on the input.
3.2.1. Current Implementation
The implementation of this command consist of two phases.
Phase 1
The modifyPay
function is first supported by ModifyPayCommandParser
which implements the Parser
interface. The interface parse the arguments parameter, which was inputted by the users to form the ModifyPayCommand
object. The arguments will also have their validity checked by the method, isValidSalary
and isValidBonus
, within respective Classes before being parse into the salary and bonus column which will return an error message when the arguments did not meet the requirements.
Code Snippet of ModifyPayCommandParser
that show the parsing of input and the checking validity of the inputs:
public class ModifyPayCommandParser implements Parser<ModifyPayCommand> {
private static final double BONUS_UPPER_LIMIT = 24.0;
public ModifyPayCommand parse(String args) throws ParseException {
requireNonNull(args);
ArgumentMultimap argMultimap = ArgumentTokenizer.tokenize(args, PREFIX_SALARY, PREFIX_BONUS);
Index index;
try {
index = ParserUtil.parseIndex(argMultimap.getPreamble());
} catch (ParseException pe) {
throw new ParseException(String.format(MESSAGE_INVALID_COMMAND_FORMAT, ModifyPayCommand.MESSAGE_USAGE), pe);
}
if (!didPrefixAppearOnlyOnce(args)) {
throw new ParseException(String.format(MESSAGE_INVALID_COMMAND_FORMAT, ModifyPayCommand.MESSAGE_USAGE));
}
ModSalaryDescriptor modSalaryDescriptor = new ModSalaryDescriptor();
if (argMultimap.getValue(PREFIX_SALARY).isPresent()) {
modSalaryDescriptor.setSalary(ParserUtil.parseSalary(argMultimap.getValue(PREFIX_SALARY).get()));
}
if (argMultimap.getValue(PREFIX_BONUS).isPresent()) {
Bonus bonusInput = ParserUtil.parseBonus(argMultimap.getValue(PREFIX_BONUS).get());
double bonus = Double.parseDouble(argMultimap.getValue(PREFIX_BONUS).get());
if (bonus > BONUS_UPPER_LIMIT) {
throw new ParseException(Bonus.MESSAGE_BONUS_CONSTRAINTS);
}
modSalaryDescriptor.setBonus(bonusInput);
}
if (!modSalaryDescriptor.isAnyFieldEdited()) {
throw new ParseException(ModifyPayCommand.MESSAGE_NOT_MODIFIED);
}
return new ModifyPayCommand(index, modSalaryDescriptor);
}
private boolean didPrefixAppearOnlyOnce(String argument) {
String salaryPrefix = " " + PREFIX_SALARY.toString();
String bonusPrefix = " " + PREFIX_BONUS.toString();
return argument.indexOf(salaryPrefix) == argument.lastIndexOf(salaryPrefix)
&& argument.indexOf(bonusPrefix) == argument.lastIndexOf(bonusPrefix);
}
}
Code snippet of Salary
public static final String MESSAGE_SALARY_CONSTRAINTS =
"Salary should only contain numbers, and it should not be blank. Only a maximum of 6 whole numbers and "
+ "2 decimal place are allowed. (Max Salary store value is 999999.99)\n";
public static final String SALARY_VALIDATION_REGEX = "[%]?[-]?[0-9]{1,6}([.][0-9]{1,2})?";
public final String value;
/**
* Constructs a {@code salary}.
*
* @param salary A valid salary.
*/
public Salary(String salary) {
requireNonNull(salary);
checkArgument(isValidSalary(salary), MESSAGE_SALARY_CONSTRAINTS);
value = salary;
}
/**
* Returns true if a given string is a valid salary.
*/
public static boolean isValidSalary(String test) {
return test.matches(SALARY_VALIDATION_REGEX);
}
Code snippet of Bonus
public static final String MESSAGE_BONUS_CONSTRAINTS =
"Bonus should only contain positive numbers and maximum of 2 decimal places from 0 to 24,"
+ " and it should not be blank";
public static final String BONUS_VALIDATION_REGEX = "(([0-9]{1,7}([.][0-9]{1,2})?)|(1[0-9]{7}([.][0-9]{1,2})?)"
+ "|(2[0-3]([0-9]{1,6})([.][0-9]{1,2})?))";
public final String value;
public Bonus(String bonus) {
requireNonNull(bonus);
checkArgument(isValidBonus(bonus), MESSAGE_BONUS_CONSTRAINTS);
value = bonus;
}
/**
* Returns true if a given string is a valid bonus.
*/
public static boolean isValidBonus(String test) {
return test.matches(BONUS_VALIDATION_REGEX);
}
Phase 2
The ModifyPayCommand
is being executed in this phase. createModifiedPerson
method calls for the edited value from ModifyPayCommandParser
to check if there are values being inputted by the users. When it is found to be a null values, createModifiedPerson
will take back the original value.
Code snippet of ModifyPayCommand
which execute the createModifiedPerson
method:
private static Person createModifiedPerson(Person personToEdit,
ModSalaryDescriptor modSalaryDescriptor) throws ParseException {
assert personToEdit != null;
EmployeeId updatedEmployeeId = personToEdit.getEmployeeId();
Name updatedName = personToEdit.getName();
DateOfBirth updatedDateOfBirth = personToEdit.getDateOfBirth();
Phone updatedPhone = personToEdit.getPhone();
Email updatedEmail = personToEdit.getEmail();
Department updatedDepartment = personToEdit.getDepartment();
Position updatedPosition = personToEdit.getPosition();
Address updatedAddress = personToEdit.getAddress();
Salary updatedSalary = ParserUtil.parseSalary(typeOfSalaryMod(personToEdit, modSalaryDescriptor));
Bonus updatedBonus = ParserUtil.parseBonus(modifyBonusMonth(personToEdit, modSalaryDescriptor, updatedSalary));
Set<Tag> updatedTags = personToEdit.getTags();
return new Person(updatedEmployeeId, updatedName, updatedDateOfBirth, updatedPhone, updatedEmail,
updatedDepartment, updatedPosition, updatedAddress, updatedSalary, updatedBonus, updatedTags);
}
The result of ModifyPayCommand
is encapsulated as a CommandResult
object which is
passed back into the UI to reflect the newly modified Salary/Bonus.
The sequence diagram below illustrates the operation of modifyPay
command:
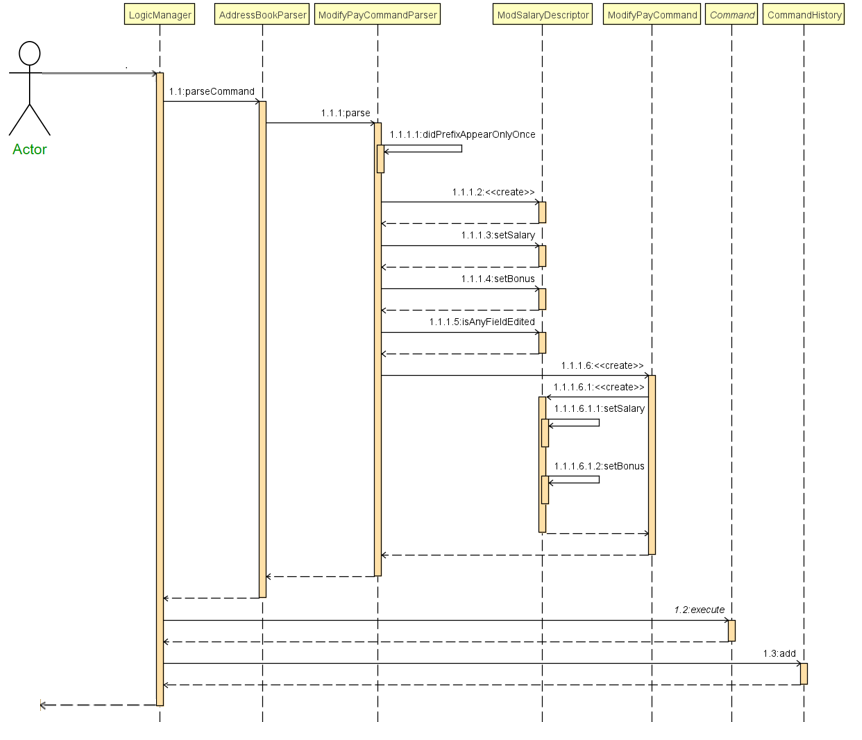
3.2.2. Employee’s Pay Report: payReport
[Upcoming in 2.0]
Generate a report to show the break down of employee’s pay such as base Salary, Bonus, Overtime Pay and CPF Contribution. [Upcoming in 2.0]
3.2.3. Design Considerations
Aspect implementation of modifyPay
command:
-
Alternative 1(current choice): A separate command to handle modification of payroll.
-
Pros: Reduce the risk of accidental editing of sensitive information
-
Cons: Additional method and prefixes are required to execute the function
-
-
Alternative 2: One command to handle all the modifications
-
Pros: Lesser Class to handle different fields
-
Cons: User may accidentally touch on sensitive data and ended up editing them
-
3.3. Recruitment Post Feature
Recruitment post
features allows HR staffs to manage internal recruitment posts easily before publishing to the public
. It allows users to do add, delete, clear, select and edit recruitment post based on 3 fields including job position,
working experience, and job description.
All logic commands come with a dedicated parser. These parsers ensure that the input conforms to the following field restrictions in phase 1 before parsing to the actual command for execution in phase 2:
Field Name | Prefix | Limitations |
---|---|---|
JOB_POSITION |
jp/ |
Job position accepts only characters. It must not be blank and should not include numbers and punctuation mark. And users are not allowed to exceed the character limit which is from 1 to 20 |
MINIMAL_YEARS_OF_WORKING_EXPERIENCE |
me/ |
Minimal years of working experience must be integers and should not be blank . And It is limited from 0 to 30 |
JOB_DESCRIPTION |
jd/ |
Job description accepts only characters and Punctuation mark including only comma, full stop, and single right quote. It must not be blank and should not include numbers. And users are not allowed to exceed the character limit which is from 1 to 200 |
3.3.1. Current Implementation for addRecruitmentPost
addRecruitmentPost
command allows a new recruitment post to be added. The sequence diagram below illustrates the
operation of addRecruitmentPost
command:

In addition, Command Exception will be thrown due to the following:
-
Invalid command format is used.
-
A duplicated recruitment field exists. Minimal 1 field should be different.
-
Fields' restrictions are not followed.
3.3.2. Current Implementation for editRecruitmentPost
editRecruitmentPost
command allows an existing recruitment post to be edited. The sequence diagram below illustrates
the operation of editRecruitmentPost
command:

In addition, Command Exception will be thrown due to the following:
-
Invalid command format is used.
-
A duplicated recruitment field exists. Minimal 1 field should be different from existing recruitment post that is about to be edited.
-
Fields' restrictions are not followed.
3.3.3. Current Implementation for selectRecruitmentPost
selectRecruitmentPost
command allows users to select an existing recruitment post based on recruit post index number
displayed in the recruitment post list panel. The sequence diagram below illustrates the operation
of selectRecruitmentPost
command:

In addition, Command Exception will be thrown due to the following:
-
Invalid command format is used.
-
Recruitment post list is empty.
-
Invalid index number is used.
3.3.4. Current Implementation for deleteRecruitmentPost
deleteRecruitmentPost
command allows users to delete an existing recruitment post based on recruit post index number
displayed in the recruitment post list panel. The sequence diagram below illustrates the operation
of deleteRecruitmentPost
command:

In addition, Command Exception will be thrown due to the following:
-
Invalid command format is used.
-
Recruitment post list is empty.
-
Invalid index number is used.
3.3.5. Future Implementation for publishRecruitmentPost
[Upcoming in 2.0]
publishRecruitmentPost
command allows users to publish an existing recruitment post to job portal such as job street
website. [Upcoming in 2.0]
3.3.6. Design Considerations for Recruitment Post Feature
Aspect implementation of all Recruitment Post Commands
-
Alternative 1 (current choice): Have separate commands to manage recruitment posts.
-
Pros: Individual command is easier for user to use and easier for developer to debug and test.
-
Cons: More methods and implementation is required.
-
-
Alternative 2: Have a single command to manage recruitment posts.
-
Pros: Lesser methods and implementation is required.
-
Cons: The command could be too complicated for users to use as more fields would be needed.
-
3.4. Schedule Feature
Schedule
command helps the HR admins to schedule employee’s work and leave schedules in the company.
A person can have multiple schedules. Type field describes whether the schedule is a work or a leave.
Below is the schedule class diagram.
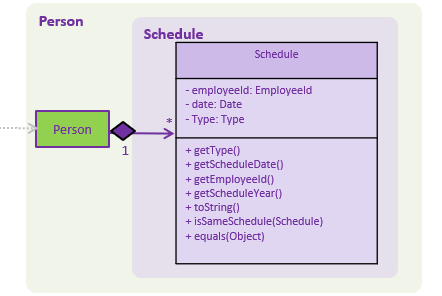
For each schedule commands, it comes with a dedicated schedule parser.
First, the schedule parsers ensure that the prefix required are all present with !arePrefixesPresent
.
...
private static boolean arePrefixesPresent(ArgumentMultimap argumentMultimap, Prefix... prefixes) {
return Stream.of(prefixes).allMatch(prefix -> argumentMultimap.getValue(prefix).isPresent());
}
...
The parsers validate field inputs by creating a new Type
, Date
, EmployeeId
and Schedule
object.
...
Type type = ParserUtil.parseStatus(argMultimap.getValue(PREFIX_SCHEDULE_TYPE).get());
Date date = ParserUtil.parseDate(argMultimap.getValue(PREFIX_SCHEDULE_DATE).get());
EmployeeId id = ParserUtil.parseEmployeeId(argMultimap.getValue(PREFIX_EMPLOYEEID).get());
Schedule schedule = new Schedule(id, type, date);
...
The objects will throw exception
if it does not conform to the following field restrictions.
Field Name | Prefix | Limitations |
---|---|---|
EMPLOYEEID |
id/ |
Employee Id should only contain exactly 6 numbers. |
DATE |
d/ |
Date must be a valid date in the calendar DD/MM/YYYY]. Year must also fall into the range of 2000-2099. Leading 0s can be omitted in day and month field. You are not allowed to schedule for dates that have past today’s date. |
TYPE |
t/ |
Type can be either WORK or LEAVE only, case not sensitive. |
3.4.1. Add Schedule
addSchedule
command allows a new work or leave schedule to be added.
Shown below is the normal flow of the sequence diagram for adding a new schedule.
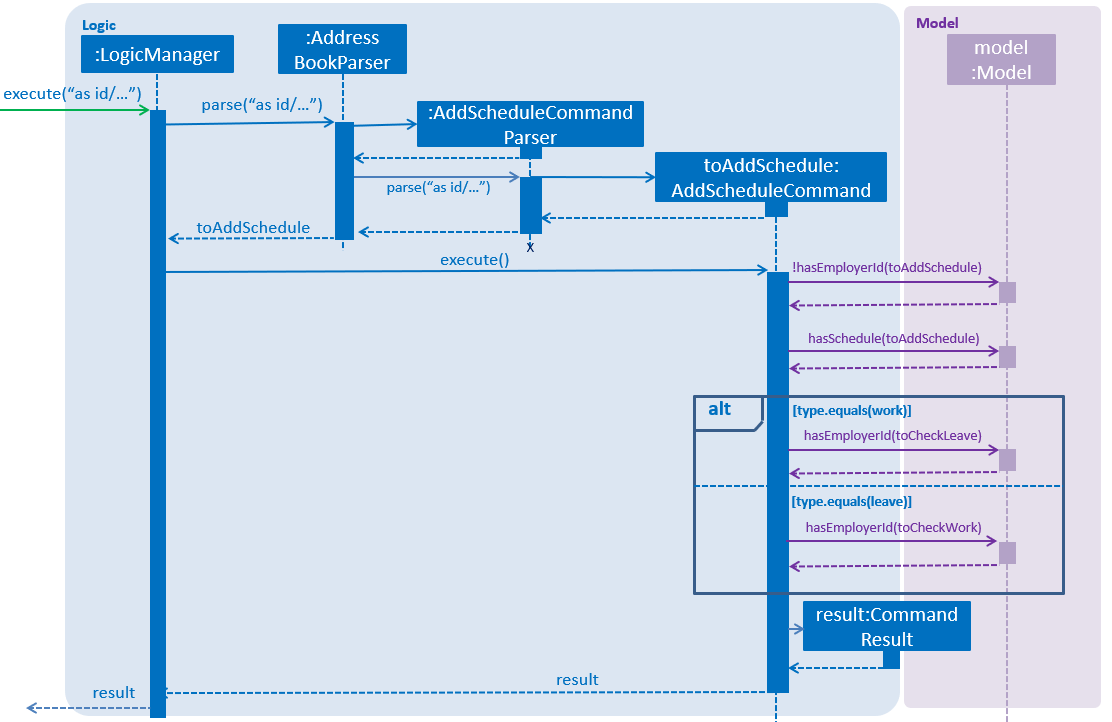
In addition, Command Exception will be thrown due to the following:
-
Employee Id of the schedule to be added is not found in address book.
-
A duplicated schedule exists.
-
User wants to add a work schedule, but a leave schedule exists on same date.
-
User wants to add leave schedule, but a work schedule exists on same date.
The AddScheduleParser
parses a Schedule
object to the AddWorks
command.
AddWorks
command looks at the Type
inside the Schedule
.
Type type = toAddSchedule.getType();
If the Type
is equals to leave and work is not scheduled on the same date model.hasSchedule(toCheckWork)
,
no exception will be thrown.
...
if (type.equals(leave)) {
Schedule toCheckWork = new Schedule(toAddSchedule.getEmployeeId(), work,
toAddSchedule.getScheduleDate());
if (model.hasSchedule(toCheckWork)) {
throw new CommandException(MESSAGE_HAS_WORK);
}
...
And it adds the schedule and commit to the storage.
...
model.addSchedule(toAddSchedule);
model.commitScheduleList();
...
3.4.2. Delete Schedule
deleteSchedule
command allows a schedule to be deleted.
Shown below is the normal flow of the sequence diagram for adding a new schedule.
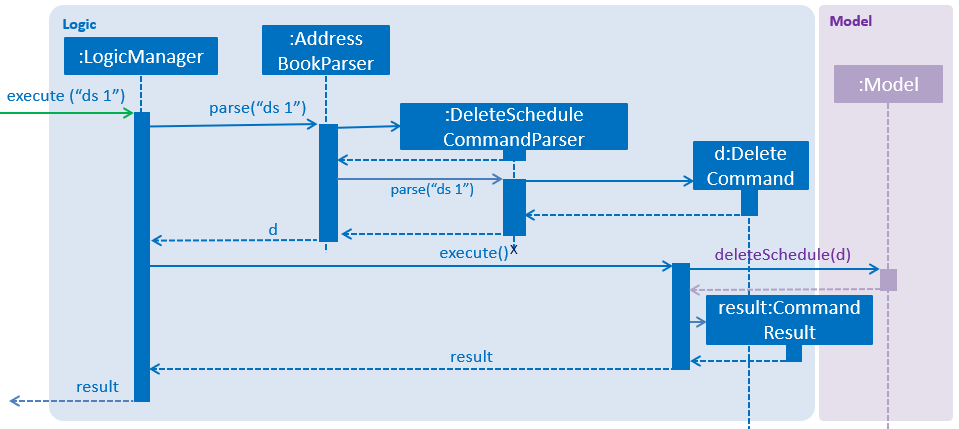
In addition, Command Exception will be thrown due to the following:
-
Index parsed is an invalid index in the ScheduleList panel.
3.4.3. Add Works
addWorks
command allows multiple work schedules to be added based on
the employees shown in the employee’s panel.
Shown below is the normal flow of the sequence diagram for adding a working schedule.
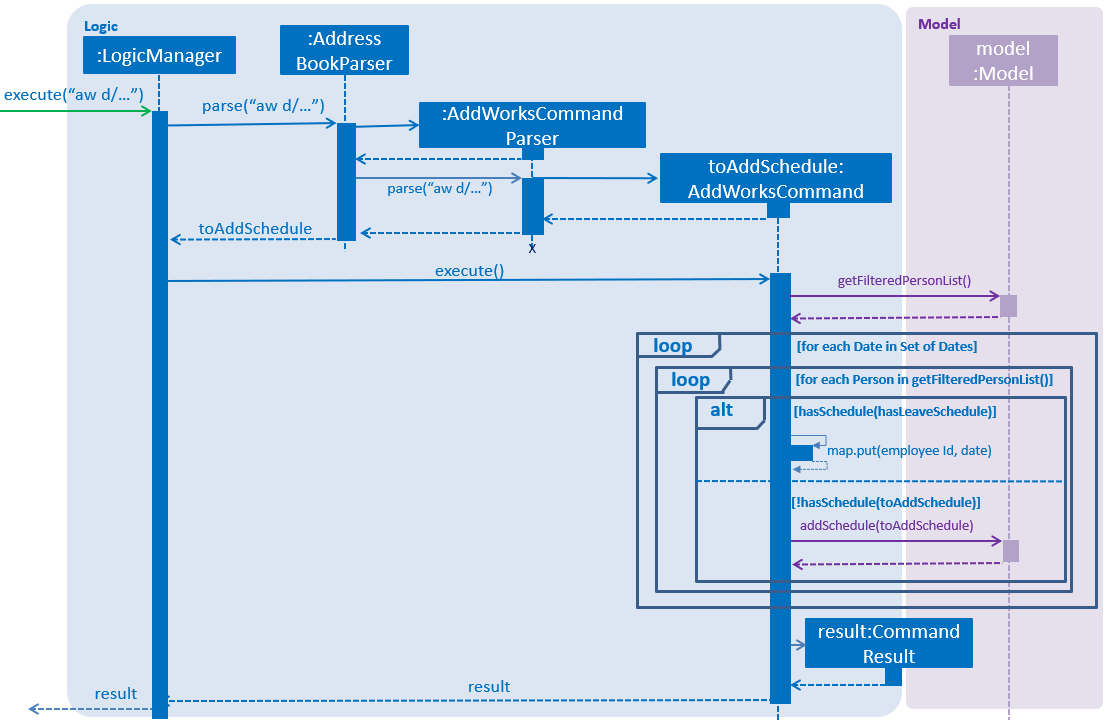
In addition, Command Exception will be thrown due to the following:
-
Size of the filtered person list is 0. This happens when there are no employees shown.
-
Commit is false. This happens either when all employees have been added work, or some had leave on the same day.
The AddWorksParser
parses a set of dates Set<Date>
to be scheduled work to the AddWorks
command.
For each date specified by the user in the set of dates for (Date date :setOfDates)
,
it will be checked by for each observable employee in the employee list for (Person person : model.getFilteredPersonList())
for the possibility of scheduling work.
No schedules will be created if a duplicate schedule model.hasSchedule(toAddSchedule)
or existing work schedule model.hasSchedule(hasLeaveSchedule)
is found on that date.
...
for (Date date :setOfDates) {
for (Person person : model.getFilteredPersonList()) {
Schedule toAddSchedule = new Schedule(person.getEmployeeId(), work , date);
Schedule hasLeaveSchedule = new Schedule(person.getEmployeeId(), leave , date);
if (model.hasSchedule(hasLeaveSchedule)) {
employeeIdMapToLeaves.put(person.getEmployeeId(), date);
} else if (!model.hasSchedule(toAddSchedule)) {
commit = true;
model.addSchedule(toAddSchedule);
}
}
}
...
3.4.4. Delete Works
deleteWorks
command allows multiple work schedules to be deleted based on
the employees shown in the employee’s panel.
Shown below is the normal flow of the sequence diagram for deleting multiple work schedules.
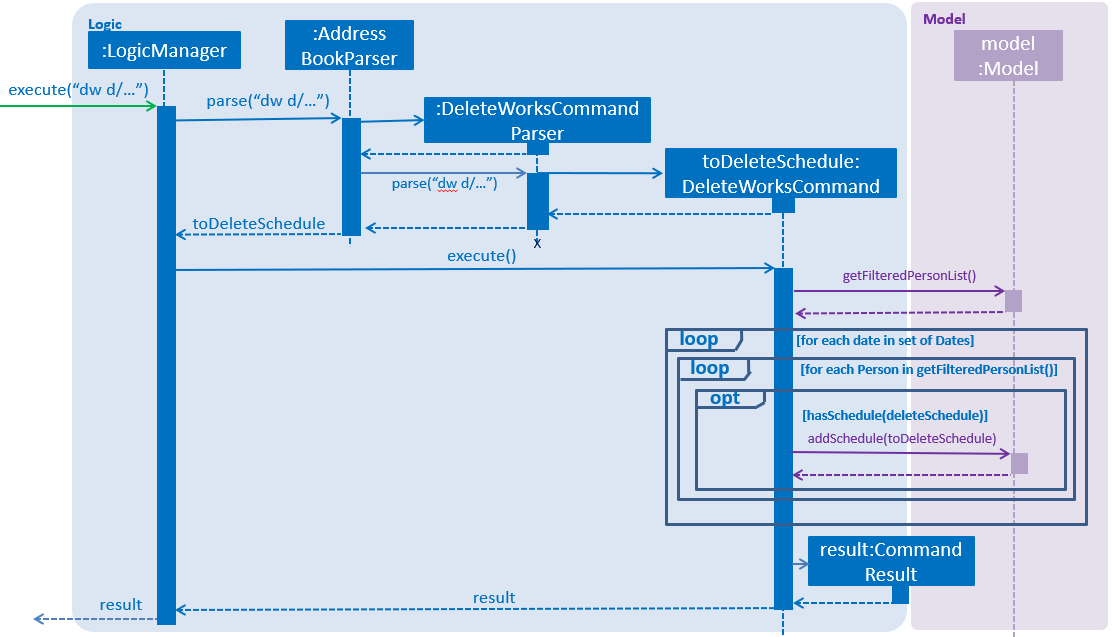
In addition, Command Exception will be thrown due to the following:
-
Size of the filtered person list is 0. This happens when there are no employees shown.
The deleteWorksParser
parses a set of dates Set<Date>
to be deleted work to the DeleteWorks
command.
For each date specified by the user in the set of dates for (Date date :setOfDates)
,
it will be checked by for each observable employee in the employee list for (Person person : model.getFilteredPersonList())
for the possibility of deleting work. Work schedule will be deleted model.deleteSchedule(toDeleteSchedule)
if found on that date model.hasSchedule(toDeleteSchedule)
.
...
for (Date date : setOfDates) {
for (Person person : model.getFilteredPersonList()) {
Schedule toDeleteSchedule = new Schedule(person.getEmployeeId(), work , date);
if (model.hasSchedule(toDeleteSchedule)) {
commit = true;
model.deleteSchedule(toDeleteSchedule);
}
}
}
...
3.4.5. Add Leaves
addLeaves
command allows multiple leave schedules to be added based on
the employees shown in the employee’s panel.
Shown below is the normal flow of the sequence diagram for adding a leave schedule.
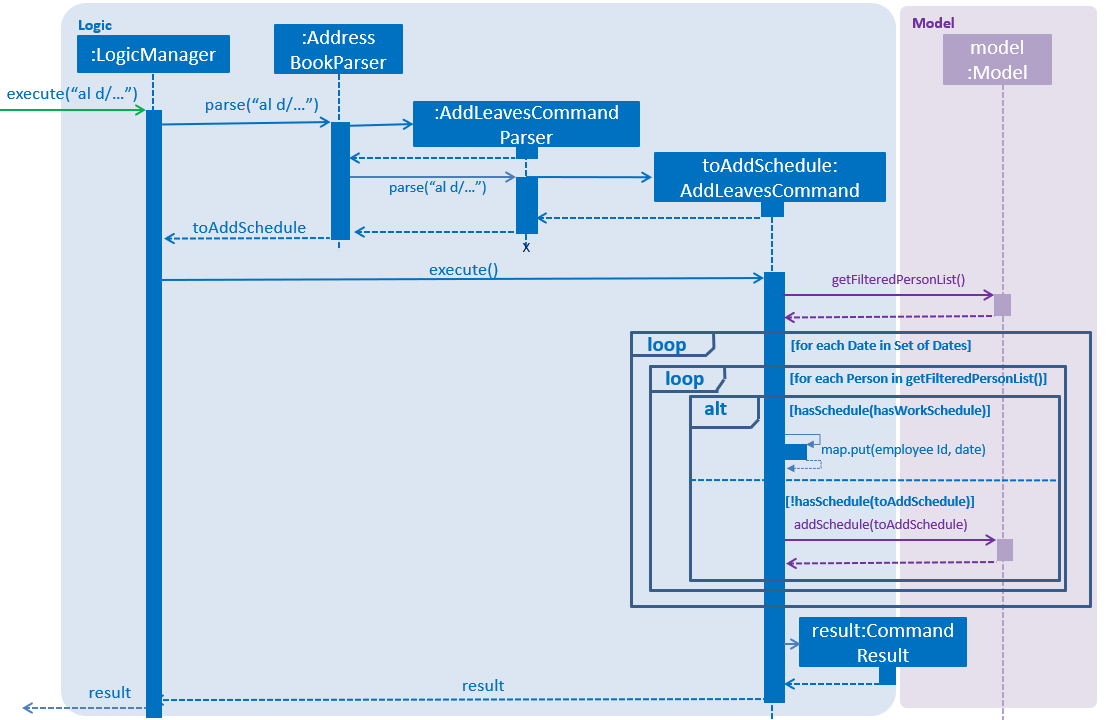
In addition, Command Exception will be thrown due to the following:
-
Size of the filtered person list is 0. This happens when there are no employees shown.
-
Commit is false. This happens either when all employees have been added work, or some had leave on the same day.
3.4.6. Delete Leaves
deleteLeaves
command allows multiple leave schedules to be deleted based on
the employees shown in the employee’s panel.
Shown below is the normal flow of the sequence diagram for deleting multiple leave schedules.
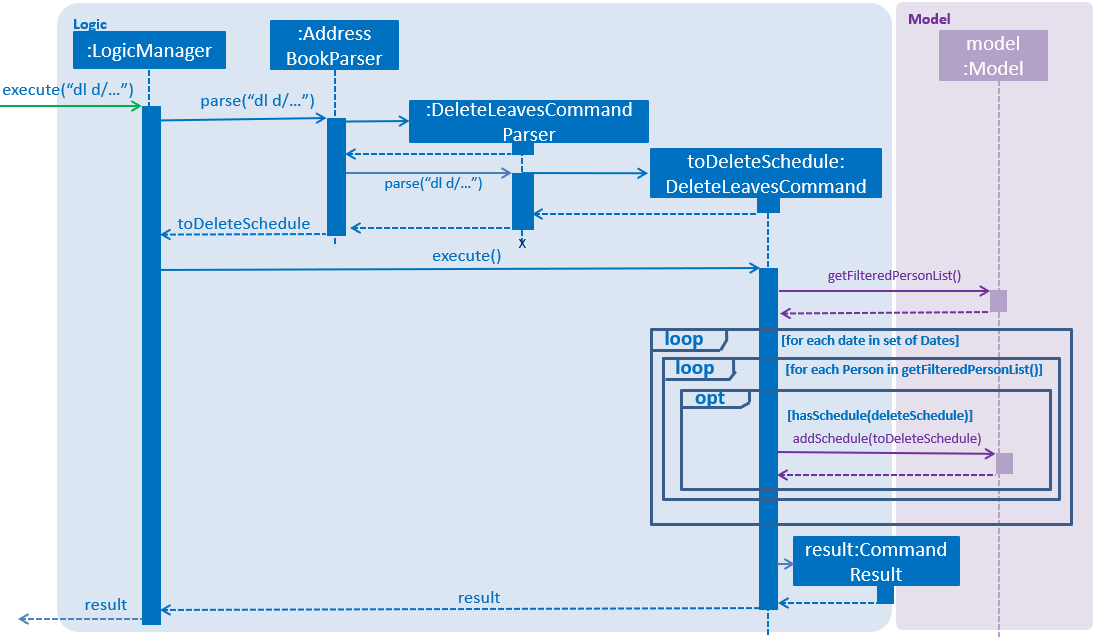
In addition, Command Exception will be thrown due to the following:
-
Size of the filtered person list is 0. This happens when there is no employees shown.
3.4.7. Calculate Leaves
calculateLeaves
command allows user to calculate leaves taken for the specified employee id and year.
Shown below is the normal flow of the sequence diagram for calculating leaves.
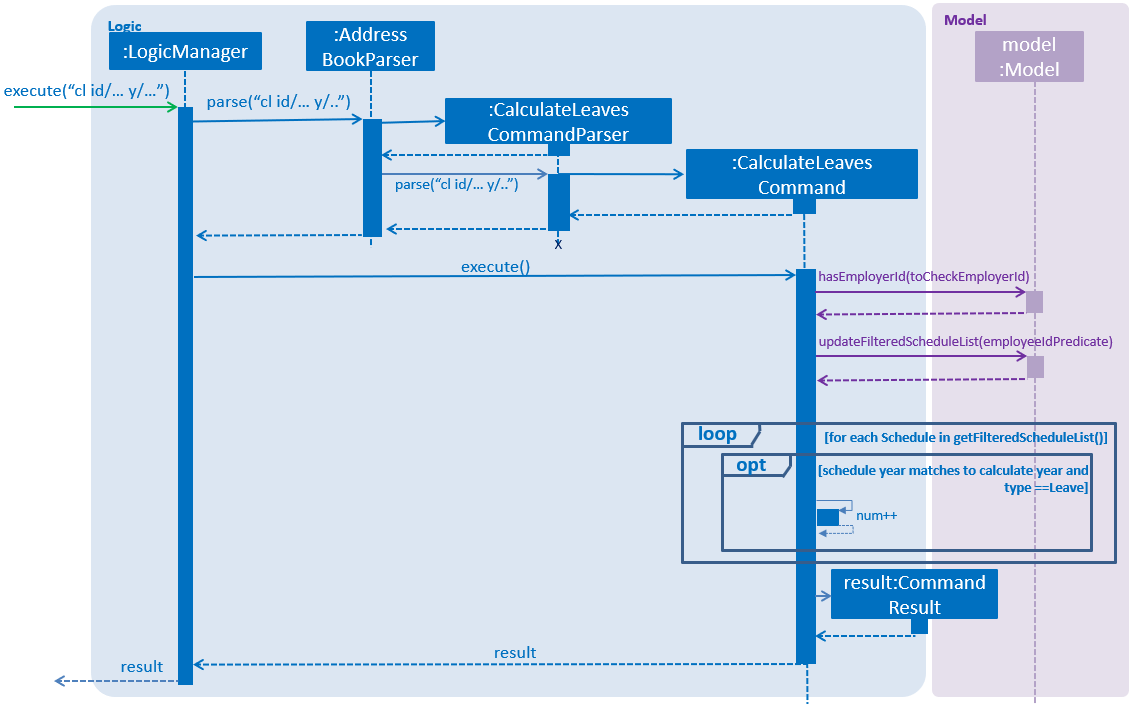
In addition, Command Exception will be thrown due to the following:
-
Employee Id to be calculated is not found in address book.
-
Employee Id has not taken any leaves in the specified year.
The CalculateLeavesCommandParser
parses a EmployeeId
and Year
object
to the CalculateLeaves
command.
CalculateLeaves
filters the schedule list to show only schedules that
contains the employeeId
to be calculated.
List<String> employeeIdList = new ArrayList<>();
employeeIdList.add(employeeId.value);
EmployeeIdScheduleContainsKeywordsPredicate employeeIdPredicate =
new EmployeeIdScheduleContainsKeywordsPredicate(employeeIdList);
model.updateFilteredScheduleList(employeeIdPredicate);
For each schedule in the filtered schedule list for (Schedule schedule : model.getFilteredScheduleList()
if the leave and year matches the one to be calculated, it
increments the number of leaves numLeaves
.
...
for (Schedule schedule : model.getFilteredScheduleList()) {
if (schedule.getScheduleYear().equals(year.toString())
&& schedule.getType().toString().equals(Type.LEAVE)) {
numLeaves++;
}
}
...
Finally it returns the calculated leave.
CommandResult(String.format(MESSAGE_SUCCESS, employeeId, year, numLeaves))
3.4.8. Send Schedule: sendSchedule
[Upcoming in 2.0]
Send schedules to the employee for calendar import using the Employee’s email address. [Upcoming in 2.0]
3.4.9. Design considerations for Schedule Features
Aspect: Command
-
Alternative 1 (current choice): Have separate commands to add work/leave schedules.
-
Pros: Easier for the user to use, without the need to specify the type of schedule.
-
Cons: More codes needed, but easier for user to schedule for multiple employees with multiple dates.
-
-
Alternative 2: Have a single command to add work/leave schedule.
-
Pros: Easier to code.
-
Cons: Requires user to specify type of schedule. Can only schedule 1 at a time.
-
Aspect: Storage
-
Alternative 1 (current choice): Have schedules stored in a separate XML file.
-
Pros: Easier to manage scalability issues as schedules grow in numbers. Possibility of having a database for schedule in future.
-
Cons: More codes needed. Extra time is needed to delete schedules when employee is deleted.
-
-
Alternative 2: Have schedules stored in the same XML file as addressbook.xml
-
Pros: Pros: Easier to implement, as only an additional field is required by modeling it as a set of schedules.
-
Cons: Very hard to manage large number of schedules, or add additional fields to describe the schedule.
-
3.5. Add Expenses
The expenses feature contains to two commands.
-
The command
addExpenses
allows the user to add expenses for each employee.
3.5.1. Current Implementation for addExpenses
command
Phase 1
The addExpenses
command is handled by AddExpensesCommandParser
.
The AddExpensesCommandParser
implements the Parser
interface, takes in input from the user as a form of argument,
The AddExpensesCommandParser
will then check if user input contains too many prefixes or check for multiple entries of
same prefix.
Next, the AddExpensesCommandParser
will compute the value of expensesAmount
to be added by summing the
the values of travelExpenses
, medicalExpenses
and miscellaneousExpenses
. The AddExpensesCommandParser
will then
create the Expenses
and EditExpensesDescriptor
objects. Other classes will handle validation of the input, check if
it has the correct input format.
The five methods that helps to valid the input are:
-
isValidEmployeeId
fromEmployeeId
class -
isValidExpensesAmount
fromExpensesAmount
class -
isValidTravelExpenses
fromTravelExpenses
class -
isValidMedicalExpenses
fromMedicalExpenses
class -
isValidMiscellaneousExpenses
fromMiscellaneousExpenses
class
If the input does not comply with the required format, an error message will be shown to the user. |
Code snippet of isValidEmployeeId
that shows the checking of the input compliance:
public static final String EMPLOYE_EXPENSES_ID_VALIDATION_REGEX = "\\d{3,}";
/**
* Returns true if a given string is a valid Employee Expenses Id.
*/
public static boolean isValidEmployeeId(String test) {
return test.matches(EMPLOYE_EXPENSES_ID_VALIDATION_REGEX);
}
Code snippet of isValidExpensesAmount
that shows the checking of the input compliance:
public static final String EMPLOYE_EXPENSES_AMOUNT_VALIDATION_REGEX = "[-]?[0-9]+(.[0-9]{0,2})?";
/**
* Returns true if a given string is a valid Expenses Amount.
*/
public static boolean isValidExpensesAmount(String test) {
return test.matches(EMPLOYE_EXPENSES_AMOUNT_VALIDATION_REGEX);
}
Phase 2
The AddExpensesCommand
will take in the Expenses
and EditExpensesDescriptor
objects.
The AddExpensesCommand
will first check if there exists a person in the person list that has the same employeeId as
Expenses
object using the condition (!model.hasEmployeeId(toCheckEmployeeId)
.
The AddExpensesCommand
will next check if there exists an expenses in the expenses list that has the same employeeId
as Expenses
object using the condition (!model.hasExpenses(toAddExpenses)
.
Code snippet of condition that checks the person and expenses list for person and expenses with same employeeId:
if (!model.hasEmployeeId(toCheckEmployeeId)) {
throw new CommandException(MESSAGE_EMPLOYEE_ID_NOT_FOUND);
} else if (!model.hasExpenses(toAddExpenses)) {
-
If Expenses List don’t have expenses with same EmployeeId,
-
The
AddExpensesCommand
will then check if any of the fields will contains negative value or exceed the limit. An exception will be thrown if at least one of the fields contains negative value or exceed the limit. -
The
AddExpensesCommand
will then add the expenses into CHRS.
-
Code snippet of If Expenses List don’t have expenses with same EmployeeId
else if (!model.hasExpenses(toAddExpenses)) {
if (Double.parseDouble(toAddExpenses.getExpensesAmount().toString()) < 0
|| Double.parseDouble(toAddExpenses.getTravelExpenses().toString()) < 0
|| Double.parseDouble(toAddExpenses.getMedicalExpenses().toString()) < 0
|| Double.parseDouble(toAddExpenses.getMiscellaneousExpenses().toString()) < 0) {
throw new CommandException(MESSAGE_NEGATIVE_LEFTOVER);
} else if (Double.parseDouble(toAddExpenses.getExpensesAmount().toString()) > MAX_TOTAL_EXPENSES
|| Double.parseDouble(toAddExpenses.getTravelExpenses().toString()) > MAX_EXPENSES_AMOUNT
|| Double.parseDouble(toAddExpenses.getMedicalExpenses().toString()) > MAX_EXPENSES_AMOUNT
|| Double.parseDouble(toAddExpenses.getMiscellaneousExpenses().toString()) > MAX_EXPENSES_AMOUNT
) {
throw new CommandException(MESSAGE_VALUE_OVER_LIMIT);
} else if (Double.parseDouble(toAddExpenses.getExpensesAmount().toString()) >= 0
&& Double.parseDouble(toAddExpenses.getTravelExpenses().toString()) >= 0
&& Double.parseDouble(toAddExpenses.getMedicalExpenses().toString()) >= 0
&& Double.parseDouble(toAddExpenses.getMiscellaneousExpenses().toString()) >= 0
&& Double.parseDouble(toAddExpenses.getExpensesAmount().toString()) <= MAX_TOTAL_EXPENSES
&& Double.parseDouble(toAddExpenses.getTravelExpenses().toString()) <= MAX_EXPENSES_AMOUNT
&& Double.parseDouble(toAddExpenses.getMedicalExpenses().toString()) <= MAX_EXPENSES_AMOUNT
&& Double.parseDouble(toAddExpenses.getMiscellaneousExpenses().toString()) <= MAX_EXPENSES_AMOUNT
) {
model.addExpenses(toAddExpenses);
model.commitExpensesList();
messageToShow = MESSAGE_SUCCESS;
}
-
If Expenses List have expenses with same EmployeeId,
-
The
AddExpensesCommand
will create anexpensesToEdit
object TheAddExpensesCommand
will then callcreateEditedExpenses
method withexpensesToEdit
andEditExpensesDescriptor
object as parameters.
-
Code snippet creating expensesToEdit
object and calling createdEditedExpenses
method
else if (model.hasExpenses(toAddExpenses)) {
EmployeeIdExpensesContainsKeywordsPredicate predicatEmployeeId;
List<String> employeeIdList = new ArrayList<>();
List<Expenses> lastShownListExpenses;
employeeIdList.add(toCheckEmployeeId.getEmployeeId().value);
predicatEmployeeId = new EmployeeIdExpensesContainsKeywordsPredicate(employeeIdList);
model.updateFilteredExpensesList(predicatEmployeeId);
lastShownListExpenses = model.getFilteredExpensesList();
Expenses expensesToEdit = lastShownListExpenses.get(0);
Expenses editedExpenses = createEditedExpenses(expensesToEdit, editExpensesDescriptor);
-
The
createEditedExpenses
method will callmodifyExpensesAmount
,modifyTravelExpenses
,modifyMedicalExpenses
,modifyMiscellaneousExpenses
, methods withexpensesToEdit
andEditExpensesDescriptor
object as parameter.
Code snippet createdEditedExpenses
method
private Expenses createEditedExpenses(Expenses expensesToEdit, EditExpensesDescriptor
editExpensesDescriptor) {
assert expensesToEdit != null;
ExpensesAmount updatedExpensesAmount = null;
TravelExpenses updatedTravelExpenses = null;
MedicalExpenses updatedMedicalExpenses = null;
MiscellaneousExpenses updatedMiscellaneousExpenses = null;
EmployeeId updatedEmployeeId = expensesToEdit.getEmployeeId();
try {
updatedExpensesAmount = ParserUtil.parseExpensesAmount(modifyExpensesAmount(expensesToEdit,
editExpensesDescriptor));
updatedTravelExpenses = ParserUtil.parseTravelExpenses(modifyTravelExpenses(expensesToEdit,
editExpensesDescriptor));
updatedMedicalExpenses = ParserUtil.parseMedicalExpenses(modifyMedicalExpenses(expensesToEdit,
editExpensesDescriptor));
updatedMiscellaneousExpenses = ParserUtil.parseMiscellaneousExpenses(modifyMiscellaneousExpenses(
expensesToEdit, editExpensesDescriptor));
} catch (ParseException pe) {
pe.printStackTrace();
}
return new Expenses(updatedEmployeeId, updatedExpensesAmount, updatedTravelExpenses, updatedMedicalExpenses,
updatedMiscellaneousExpenses);
}
-
Each method will edit their respective field from
expensesToEdit
with the same field fromEditExpensesDescriptor
. The method will set isNegativeLeftover to be true and set isOverLimit to be true if the values is below 0 or exceed max value.
Code snippet modifyExpensesAmount
method, one of the methods being called
private String modifyExpensesAmount (Expenses expensesToEdit, EditExpensesDescriptor
editExpensesDescriptor) {
NumberFormat formatter = new DecimalFormat("#0.00");
String newExpensesAmount = expensesToEdit.getExpensesAmount().toString();
double updateExpensesAmount = Double.parseDouble(newExpensesAmount);
String change = editExpensesDescriptor.getExpensesAmount().toString().replaceAll("[^0-9.-]",
"");
updateExpensesAmount += Double.parseDouble(change);
if (updateExpensesAmount < 0) {
setIsNegativeLeftover(true);
} else if (updateExpensesAmount > MAX_TOTAL_EXPENSES) {
setIsOverLimit(true);
} else if (updateExpensesAmount >= 0) {
newExpensesAmount = String.valueOf(formatter.format(updateExpensesAmount));
}
return newExpensesAmount;
}
-
The
AddExpensesCommand
will check for isNegativeLeftover and isOverLimit. If both are false, theAddExpensesCommand
will then update the updated expenses into CHRS.
Code snippet of isNegativeLeftover and isOverLimit check
if (getIsNegativeLeftover()) {
throw new CommandException(MESSAGE_NEGATIVE_LEFTOVER);
} else if (getIsOverLimit()) {
throw new CommandException(MESSAGE_VALUE_OVER_LIMIT);
} else if (!getIsNegativeLeftover() && !getIsOverLimit()) {
messageToShow = MESSAGE_SUCCESS;
model.updateExpenses(expensesToEdit, editedExpenses);
model.commitExpensesList();
}
model.updateFilteredExpensesList(PREDICATE_SHOW_ALL_EXPENSES);
}
The sequence diagram below illustrates the operation of the AddExpensesCommand
:

3.5.2. Design Considerations
Aspect implementation of AddExpenses
command:
-
Alternative 1 (current choice): Have a single command to add and modify expenses.
-
Pros: User isn’t require to ensure that there exist an expenses for the employee before modifying the expenses
-
Cons: Have to handle more conditions and possibly more prone to bugs.
-
-
Alternative 2: Have separate commands to add or modify expenses.
-
Pros: User won’t have the possibly of mixing up if they want to add new expenses or modify old expenses.
-
Cons: Requires user to know more commands.
-
Aspect: Expenses List
Storage
-
Alternative 1 (current choice): Have expenses stored in XML format.
-
Pros: Easier to read, code and test XML files.
-
Cons: Can’t compute or tabulate data from file.
-
-
Alternative 2: Have schedules stored in CSV format.
-
Pros: More efficient way of storing tabular data like expenses
-
Cons: Harder to read as compared to XML file.
-
3.5.3. Expenses Report: expensesReport
[Upcoming in 2.0]
Generates an expenses report with the total expenses amount claimed and have not been claimed for a year [Upcoming in 2.0]
3.6. Filter Feature
The command filter
allows the user to filter employees based on their respective department and/or position within the company.
3.6.1. Current Implementation
The implementation of this command is separated into two phases.
Phase 1
The filter
command’s mechanism is facilitated by FilterCommandParser
.
The FilterCommandParser
implements the Parser
interface, parses the arguments parameter, which is the input from the user, and creates a new FilterCommand
object.
Apart from parsing the input, the FilterCommandParser
also checks whether the input complies with the required format.
The checking of input compliance is assisted by a helper method, didPrefixesAppearOnlyOnce
, which checks whether the department prefix and/or position prefix appears only once.
If the input does not comply with the required format, an error message will be shown to the user. |
Code snippet of the parse
method within FilterCommandParser
showing the checks for input compliance:
public FilterCommand parse(String args) throws ParseException {
...
...
ArgumentMultimap argMultimap = ArgumentTokenizer.tokenize(args, PREFIX_DEPARTMENT, PREFIX_POSITION);
if (trimmedArgs.isEmpty() || (!argMultimap.getValue(PREFIX_DEPARTMENT).isPresent()
&& !argMultimap.getValue(PREFIX_POSITION).isPresent()) || !didPrefixesAppearOnlyOnce(trimmedArgs)
|| !ACCEPTED_ORDERS.contains(sortOrder)) {
throw new ParseException(String.format(MESSAGE_INVALID_COMMAND_FORMAT, FilterCommand.MESSAGE_USAGE));
}
...
...
}
Code snippet of the helper method didPrefixesAppearOnlyOnce
:
public boolean didPrefixesAppearOnlyOnce(String argument) {
String departmentPrefix = " " + PREFIX_DEPARTMENT.toString();
String positionPrefix = " " + PREFIX_POSITION.toString();
return argument.indexOf(departmentPrefix) == argument.lastIndexOf(departmentPrefix)
&& argument.indexOf(positionPrefix) == argument.lastIndexOf(positionPrefix);
}
If the input complies with the required format, further checks will be performed on the keywords to ensure that the keywords are valid.
This keywords checking is completed by the processDepartmentKeywords
and processPositionKeywords
methods that are both within the FilterCommandParser
class.
This guide will focus on explaining how processDepartmentKeywords
work instead of both as the methods processDepartmentKeywords
and processPositionKeywords
are similar.
Code snippet of the parse
method in FilterCommandParser
calling processDepartmentKeywords
method:
public FilterCommand parse(String args) throws ParseException {
...
...
if (!argMultimap.getValue(PREFIX_DEPARTMENT).isPresent()) {
filterCommand.setIsDepartmentPrefixPresent(false);
} else if (argMultimap.getValue(PREFIX_DEPARTMENT).isPresent()
&& !processDepartmentKeywords(argMultimap, filterCommand)) {
throw new ParseException(Department.MESSAGE_DEPARTMENT_KEYWORD_CONSTRAINTS);
}
...
...
}
Code snippet of processDepartmentKeywords
helper method:
public boolean processDepartmentKeywords(ArgumentMultimap argMultimap, FilterCommand command) {
String trimmedDepartment = (argMultimap.getValue(PREFIX_DEPARTMENT).get().trim());
String[] departmentKeywords = trimmedDepartment.split("\\s+");
return validityCheckForDepartments(command, departmentKeywords);
}
The processDepartmentKeywords
is further assisted by the validityCheckForDepartments
method which calls another method areDepartmentKeywordsValid
to check whether the given keywords comply with the DEPARTMENT_KEYWORD_VALIDATION_REGEX
.
If the keywords from the user are all valid, the departmentPredicate will be created and passed to the FilterCommand
object through a setter.
The DEPARTMENT_KEYWORD_VALIDATION_REGEX is a regular expression that accepts only spaces and case-insensitive alphabets.
It only allows 1 to 30 characters per keyword.
|
Code snippet of validityCheckForDepartments
and areDepartmentKeywordsValid
methods in FilterCommandParser
class:
public boolean validityCheckForDepartments(FilterCommand command, String[] keywords) {
if (!areDepartmentKeywordsValid(keywords)) {
return false;
}
command.setIsDepartmentPrefixPresent(true);
command.setDepartmentPredicate(new DepartmentContainsKeywordsPredicate(Arrays.asList(keywords)));
return true;
}
public boolean areDepartmentKeywordsValid(String[] keywords) {
for (String keyword: keywords) {
if (!keyword.matches(DEPARTMENT_KEYWORD_VALIDATION_REGEX)) {
return false;
}
}
return true;
}
Phase 2
The FilterCommand
is being executed in this phase.
FilterCommand
first checks which prefix(es) is(are) present prior to calling the updateFilteredPersonList
method, which updates filteredPersonList
with the employees of the relevant department(s) and/or position(s) that matches the keyword(s) input from the user.
Additionally, the methods updateFilteredExpensesList
and updateFilteredScheduleList
will also be called to update the filteredExpensesList
and filteredScheduleList
respectively to show only the matched employees' expenses and schedules.
If the keywords input from the user results in 0 person found. The CLI will show the user a list of currently available department(s) and/or position(s) in the PersonList.
The listing of available department(s) and/or position(s) depends on the presence of the prefix. Example: If filter asc d/hello results in 0 person found, the CLI will list all the currently available department(s) in CHRS.
|
Code snippet of FilterCommand
that checks which prefix is present prior to updating filteredPersonList
, filteredExpensesList
and filteredScheduleList
:
public class FilterCommand extends Command {
...
...
@Override
public CommandResult execute(Model model, CommandHistory history) {
requireNonNull(model);
String allAvailableDepartments = listAvailableDepartments(model);
String allAvailablePositions = listAvailablePositions(model);
if (isDepartmentPrefixPresent && !isPositionPrefixPresent) {
model.updateFilteredPersonList(departmentPredicate, sortOrder);
} else if (isPositionPrefixPresent && !isDepartmentPrefixPresent) {
model.updateFilteredPersonList(positionPredicate, sortOrder);
} else if (isDepartmentPrefixPresent && isPositionPrefixPresent) {
model.updateFilteredPersonList(departmentPredicate.and(positionPredicate), sortOrder);
}
EmployeeIdExpensesContainsKeywordsPredicate expensesPredicate = generateEmployeeIdExpensesPredicate(model);
EmployeeIdScheduleContainsKeywordsPredicate schedulePredicate = generateEmployeeIdSchedulePredicate(model);
model.updateFilteredExpensesList(expensesPredicate);
model.updateFilteredScheduleList(schedulePredicate);
return new CommandResult(feedbackToUser(model, allAvailableDepartments, allAvailablePositions));
}
...
...
}
The result of the execution of FilterCommand
is then encapsulated as a CommandResult
object which is returned to the LogicManager
.
During the execution of FilterCommand
, the filteredPersonList
, filteredExpensesList
and filteredScheduleList
will be updated accordingly to display only employees, that belongs to the department(s)
and/or hold the specified position(s) as specified by the user input.
The sequence diagram below illustrates the operation of filter
command:

3.6.2. Design Considerations
Aspect: Implementation of filter
command
-
Alternative 1 (current choice): One command to handle filtering by department(s) and/or position(s)
-
Pros: Does not require several command and parser files to handle filtering of different fields
-
Cons: Additional methods required to check for presence of prefix and input validity
-
-
Alternative 2: Separate commands for filtering department and position, one for department and one for position
-
Pros: Easy to implement as it follows the existing
find
command -
Cons: More commands to create and implementation can get quite complicated when filter requires both fields
-
3.6.3. Future improvements: Keyword suggestion and auto complete feature [Upcoming in 2.0]
This feature will suggest keywords when filtering or finding employee and the keyword will be auto-completed by pressing TAB
key. [Upcoming in 2.0]
3.7. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.8, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.8. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
4. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
4.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
4.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
4.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
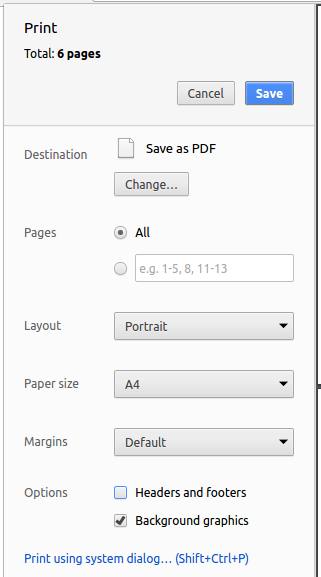
4.4. Site-wide Documentation Settings
The build.gradle
file specifies some project-specific asciidoc attributes which affects how all documentation files within this project are rendered.
Attributes left unset in the build.gradle file will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
The name of the website. If set, the name will be displayed near the top of the page. |
not set |
|
URL to the site’s repository on GitHub. Setting this will add a "View on GitHub" link in the navigation bar. |
not set |
|
Define this attribute if the project is an official SE-EDU project. This will render the SE-EDU navigation bar at the top of the page, and add some SE-EDU-specific navigation items. |
not set |
4.5. Per-file Documentation Settings
Each .adoc
file may also specify some file-specific asciidoc attributes which affects how the file is rendered.
Asciidoctor’s built-in attributes may be specified and used as well.
Attributes left unset in .adoc files will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
Site section that the document belongs to.
This will cause the associated item in the navigation bar to be highlighted.
One of: * Official SE-EDU projects only |
not set |
|
Set this attribute to remove the site navigation bar. |
not set |
4.6. Site Template
The files in docs/stylesheets
are the CSS stylesheets of the site.
You can modify them to change some properties of the site’s design.
The files in docs/templates
controls the rendering of .adoc
files into HTML5.
These template files are written in a mixture of Ruby and Slim.
Modifying the template files in |
5. Testing
5.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
5.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.address.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
5.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
6. Dev Ops
6.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
6.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
6.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
6.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
6.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
6.6. Managing Dependencies
A project often depends on third-party libraries. For example, Address Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Suggested Programming Tasks to Get Started
Suggested path for new programmers:
-
First, add small local-impact (i.e. the impact of the change does not go beyond the component) enhancements to one component at a time. Some suggestions are given in Section A.1, “Improving each component”.
-
Next, add a feature that touches multiple components to learn how to implement an end-to-end feature across all components. Section A.2, “Creating a new command:
remark
” explains how to go about adding such a feature.
A.1. Improving each component
Each individual exercise in this section is component-based (i.e. you would not need to modify the other components to get it to work).
Logic
component
Scenario: You are in charge of logic
. During dog-fooding, your team realize that it is troublesome for the user to type the whole command in order to execute a command. Your team devise some strategies to help cut down the amount of typing necessary, and one of the suggestions was to implement aliases for the command words. Your job is to implement such aliases.
Do take a look at Section 2.3, “Logic component” before attempting to modify the Logic component.
|
-
Add a shorthand equivalent alias for each of the individual commands. For example, besides typing
clear
, the user can also typec
to remove all persons in the list.
Model
component
Scenario: You are in charge of model
. One day, the logic
-in-charge approaches you for help. He wants to implement a command such that the user is able to remove a particular tag from everyone in the address book, but the model API does not support such a functionality at the moment. Your job is to implement an API method, so that your teammate can use your API to implement his command.
Do take a look at Section 2.4, “Model component” before attempting to modify the Model component.
|
-
Add a
removeTag(Tag)
method. The specified tag will be removed from everyone in the address book.
Ui
component
Scenario: You are in charge of ui
. During a beta testing session, your team is observing how the users use your address book application. You realize that one of the users occasionally tries to delete non-existent tags from a contact, because the tags all look the same visually, and the user got confused. Another user made a typing mistake in his command, but did not realize he had done so because the error message wasn’t prominent enough. A third user keeps scrolling down the list, because he keeps forgetting the index of the last person in the list. Your job is to implement improvements to the UI to solve all these problems.
Do take a look at Section 2.2, “UI component” before attempting to modify the UI component.
|
-
Use different colors for different tags inside person cards. For example,
friends
tags can be all in brown, andcolleagues
tags can be all in yellow.Before
After
-
Modify
NewResultAvailableEvent
such thatResultDisplay
can show a different style on error (currently it shows the same regardless of errors).Before
After
-
Modify the
StatusBarFooter
to show the total number of people in the address book.Before
After
Storage
component
Scenario: You are in charge of storage
. For your next project milestone, your team plans to implement a new feature of saving the address book to the cloud. However, the current implementation of the application constantly saves the address book after the execution of each command, which is not ideal if the user is working on limited internet connection. Your team decided that the application should instead save the changes to a temporary local backup file first, and only upload to the cloud after the user closes the application. Your job is to implement a backup API for the address book storage.
Do take a look at Section 2.5, “Storage component” before attempting to modify the Storage component.
|
-
Add a new method
backupAddressBook(ReadOnlyAddressBook)
, so that the address book can be saved in a fixed temporary location.
A.2. Creating a new command: remark
By creating this command, you will get a chance to learn how to implement a feature end-to-end, touching all major components of the app.
Scenario: You are a software maintainer for addressbook
, as the former developer team has moved on to new projects. The current users of your application have a list of new feature requests that they hope the software will eventually have. The most popular request is to allow adding additional comments/notes about a particular contact, by providing a flexible remark
field for each contact, rather than relying on tags alone. After designing the specification for the remark
command, you are convinced that this feature is worth implementing. Your job is to implement the remark
command.
A.2.1. Description
Edits the remark for a person specified in the INDEX
.
Format: remark INDEX r/[REMARK]
Examples:
-
remark 1 r/Likes to drink coffee.
Edits the remark for the first person toLikes to drink coffee.
-
remark 1 r/
Removes the remark for the first person.
A.2.2. Step-by-step Instructions
[Step 1] Logic: Teach the app to accept 'remark' which does nothing
Let’s start by teaching the application how to parse a remark
command. We will add the logic of remark
later.
Main:
-
Add a
RemarkCommand
that extendsCommand
. Upon execution, it should just throw anException
. -
Modify
AddressBookParser
to accept aRemarkCommand
.
Tests:
-
Add
RemarkCommandTest
that tests thatexecute()
throws an Exception. -
Add new test method to
AddressBookParserTest
, which tests that typing "remark" returns an instance ofRemarkCommand
.
[Step 2] Logic: Teach the app to accept 'remark' arguments
Let’s teach the application to parse arguments that our remark
command will accept. E.g. 1 r/Likes to drink coffee.
Main:
-
Modify
RemarkCommand
to take in anIndex
andString
and print those two parameters as the error message. -
Add
RemarkCommandParser
that knows how to parse two arguments, one index and one with prefix 'r/'. -
Modify
AddressBookParser
to use the newly implementedRemarkCommandParser
.
Tests:
-
Modify
RemarkCommandTest
to test theRemarkCommand#equals()
method. -
Add
RemarkCommandParserTest
that tests different boundary values forRemarkCommandParser
. -
Modify
AddressBookParserTest
to test that the correct command is generated according to the user input.
[Step 3] Ui: Add a placeholder for remark in PersonCard
Let’s add a placeholder on all our PersonCard
s to display a remark for each person later.
Main:
-
Add a
Label
with any random text insidePersonListCard.fxml
. -
Add FXML annotation in
PersonCard
to tie the variable to the actual label.
Tests:
-
Modify
PersonCardHandle
so that future tests can read the contents of the remark label.
[Step 4] Model: Add Remark
class
We have to properly encapsulate the remark in our Person
class. Instead of just using a String
, let’s follow the conventional class structure that the codebase already uses by adding a Remark
class.
Main:
-
Add
Remark
to model component (you can copy fromAddress
, remove the regex and change the names accordingly). -
Modify
RemarkCommand
to now take in aRemark
instead of aString
.
Tests:
-
Add test for
Remark
, to test theRemark#equals()
method.
[Step 5] Model: Modify Person
to support a Remark
field
Now we have the Remark
class, we need to actually use it inside Person
.
Main:
-
Add
getRemark()
inPerson
. -
You may assume that the user will not be able to use the
add
andedit
commands to modify the remarks field (i.e. the person will be created without a remark). -
Modify
SampleDataUtil
to add remarks for the sample data (delete youraddressBook.xml
so that the application will load the sample data when you launch it.)
[Step 6] Storage: Add Remark
field to XmlAdaptedPerson
class
We now have Remark
s for Person
s, but they will be gone when we exit the application. Let’s modify XmlAdaptedPerson
to include a Remark
field so that it will be saved.
Main:
-
Add a new Xml field for
Remark
.
Tests:
-
Fix
invalidAndValidPersonAddressBook.xml
,typicalPersonsAddressBook.xml
,validAddressBook.xml
etc., such that the XML tests will not fail due to a missing<remark>
element.
[Step 6b] Test: Add withRemark() for PersonBuilder
Since Person
can now have a Remark
, we should add a helper method to PersonBuilder
, so that users are able to create remarks when building a Person
.
Tests:
-
Add a new method
withRemark()
forPersonBuilder
. This method will create a newRemark
for the person that it is currently building. -
Try and use the method on any sample
Person
inTypicalPersons
.
[Step 7] Ui: Connect Remark
field to PersonCard
Our remark label in PersonCard
is still a placeholder. Let’s bring it to life by binding it with the actual remark
field.
Main:
-
Modify
PersonCard
's constructor to bind theRemark
field to thePerson
's remark.
Tests:
-
Modify
GuiTestAssert#assertCardDisplaysPerson(…)
so that it will compare the now-functioning remark label.
[Step 8] Logic: Implement RemarkCommand#execute()
logic
We now have everything set up… but we still can’t modify the remarks. Let’s finish it up by adding in actual logic for our remark
command.
Main:
-
Replace the logic in
RemarkCommand#execute()
(that currently just throws anException
), with the actual logic to modify the remarks of a person.
Tests:
-
Update
RemarkCommandTest
to test that theexecute()
logic works.
A.2.3. Full Solution
See this PR for the step-by-step solution.
Appendix B: Product Scope
Target user profile: Human Resource (HR) Department
Product Scope: Resolve accessibility problems and provide a centralised database for end users’ convenience
Value Proposition:
-
A centralised system with specific fields required by HR. Some fields are as follow:
Employees' salary and bonus
Employees' expenses claims, such as medical and transport
Employees' leave balance
-
Working schedule of employees (in a timetable format, GUI)
-
Recruitment post(s) to advertise the positions available within the company
-
Generation of reports such as:
Employees' claim history
Employees' salary slip
Employees' past training(s)
-
An internal messenger to enable communication between the HR department and employees
-
Function to schedule training for employees
Appendix C: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
HR Staff |
have filter functions |
search for more specific data |
|
HR Staff |
edit current employee’s data |
edit the employee’s data accordingly |
|
HR Staff |
add new employee’s data |
add new employee’s data into the system |
|
HR Staff |
delete old employee’s data |
remove employee’s details that are no longer required |
|
HR Staff (handling payroll) |
have auto calculation of pay and bonus increments |
avoid doing the calculations manually |
|
New HR Staff |
view all the available functions |
know the available functions for use in the application |
|
HR Staff (handling financial matters) |
view all the expenses claim by each employee that has yet to be claimed |
avoid keeping track of them manually |
|
HR Staff |
have a search function |
search for employee’s data easily |
|
HR Staff (handling financial matters) |
add expenses claim by employee at individual level |
keep track of employee’s expenses claim easily |
|
HR Manager |
have a function to add or remove leave/off |
keep track employee’s leave/off easily. |
|
HR Staff (handling financial matters) |
remove expenses claim by employee after the claim is completed |
keep track of employee’s expenses claim easily |
|
HR Staff (handling recruitment) |
list all the past recruitment post(s) created |
keep track of what position has already been posted for recruiting |
|
HR Staff (handling recruitment) |
add recruitment post(s) |
put up new recruitment post(s) for newly available positions |
|
HR Staff (handling recruitment) |
remove recruitment post(s) |
take down old recruitment post(s) for positions that have already been filled |
|
HR Staff (handling recruitment) |
edit recruitment post(s) |
edit currently available recruitment post(s) at any time |
|
HR Director |
have different user access |
control the information access within the system |
|
HR Manager |
generate a report of my employees' data |
have an overview of the employees |
|
HR Staff |
have departments tagged to each personnel |
search for staffs in a specific department easily |
|
HR Staff |
have shorter commands |
reduce the amount of typing required |
|
HR Staff |
have an autocomplete function |
type my commands partially and have lower risks of typing wrong commands |
|
HR Manager |
track who last made changes to the work schedule |
find out who to clarify doubts with (if any) |
|
HR Manager |
view the work schedule for each day |
find out which employees are working on which day |
|
HR Manager |
have a function to handle leave related issues |
approve/disprove employee’s leave |
|
HR Staff |
undo the last change |
correct my mistake easily |
|
HR Staff |
redo the last change |
correct my undo easily |
|
Paranoid HR Staff |
have the option to hide personally identifiable information |
lower the chances of my information being leaked or seen |
Appendix D: Use Cases
(For all use cases below, the System is the CHRS
and the Actor is the HR Staff
, unless specified otherwise)
Use case: UC01 - Employee data filter function
MSS
-
User requests to filter employees based on department(s) and/or rank/position(s)
-
CHRS output the date of the employees of the specified department(s) and/or rank(s)/position(s)
Use case ends.
Extensions
-
1a. CHRS detects an invalid department or position keyword(s).
-
1a1. CHRS informs the user that the keyword is invalid.
Use case resumes at step 1.
-
-
1b. CHRS detects that the system holds no data of any employee.
-
1b1. CHRS informs user that the system has no records of any employee data and shows the currently available department(s) and/or rank(s)/position(s) to filter by.
Use case ends.
-
-
1c. CHRS detects an invalid input format or missing details.
-
1c1. CHRS shows a message containing details of the command usage.
Use case resumes at step 1.
-
Use case: UC02 - Edit employee’s data
MSS
-
User requests to list employees
-
CHRS shows a list of employees
-
User requests to edit a specific employee’s data in the list
-
CHRS updates relevant data of the employee
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. CHRS shows an error message.
Use case resumes at step 2.
-
-
3b. CHRS detects invalid input format or missing details.
-
3b1. CHRS shows an error message containing either the details of the command usage or parameters constraints.
Use case resumes at step 2.
-
Use case: UC03 - Add employee’s data
MSS
-
User requests to add new employee’s data
-
CHRS stores the data of the employee
Use case ends.
Extensions
-
1a. CHRS detects that the employee’s data already exists.
-
1a1. CHRS informs user that the data already exists.
Use case resumes at step 1.
-
-
1b. CHRS detects invalid input format or missing details.
-
1b1. CHRS shows an error message containing either the details of the command usage or parameters constraints.
Use case resumes at step 1.
-
Use case: UC04 - Delete employee’s data
MSS
-
User requests to list employees
-
CHRS shows a list of employees
-
User requests to delete employee’s data
-
CHRS deletes the all data associated to the employee
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. CHRS shows an error message.
Use case resumes at step 2.
-
Actor: HR Staff (Handling payroll)
Use case: UC05 - Pay/Bonus increment or decrement
MSS
-
User requests to list employees
-
CHRS shows a list of employees
-
User requests to modify employee(s) pay/bonus
-
CHRS updates the pay and/or bonus of the specified employee(s)
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. CHRS shows an error message.
Use case resumes at step 2.
-
-
3b. CHRS detects invalid input format or missing details.
-
3b1. CHRS shows an error message containing either the details of the command usage or parameters constraints.
Use case resumes at step 2.
-
Use case: UC06 - View available functions or commands
MSS
-
User requests to view the available functions or commands in CHRS
-
CHRS shows the list of available functions or commands
Use case ends.
Actor: HR Staff
Use case: UC07 - List all CHRS data
MSS
-
User requests to list all CHRS data
-
CHRS shows all CHRS data
Use case ends.
Use case: UC08 - Search function
MSS
-
User requests to search for a certain employee’s data by either the name or employee ID
-
CHRS shows the data associated with the employee’s name or employee ID
Use case ends.
Extensions
-
1a. CHRS detects that requested employee’s data does not exist.
-
1a1. CHRS informs user that the data does not exist.
Use case ends.
-
-
1b. CHRS detects invalid input format or missing details.
-
1b1. CHRS shows an error message containing either the details of the command usage or parameters constraints.
Use case resumes at step 1.
-
Actor: HR Staff (Handling financial matters)
Use case: UC09 – Add expenses
MSS
-
User requests to add expenses claim by a certain employee
-
CHRS updates the expenses claim by that employee in the system
Use case ends.
Extensions
-
1a. CHRS detects that requested employee does not exist.
-
1a1. CHRS informs user that the employee does not exist.
Use case resumes at step 1.
-
-
1b. CHRS detects invalid input format or expenses exceed limit or result in negative value.
-
1b1. CHRS shows an error message containing either the details of the command usage or parameters constraints.
Use case resumes at step 1.
-
Actor: HR Staff (Handling financial matters)
Use case: UC10 - Delete expenses
MSS
-
User requests to list expenses claims
-
CHRS shows a list of expenses claims
-
User requests to delete expenses claim by a certain employee
-
CHRS deletes the expenses claim by that employee in the system
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. CHRS shows an error message.
Use case resumes at step 2.
-
Actor: HR Staff (Handling recruitment)
Use case: UC11 – Add recruitment post
MSS
-
User requests to add a new recruitment post
-
CHRS stores the details of the new recruitment post
Use case ends.
Extensions
-
1a. CHRS detects that the recruitment post already exists.
-
1a1. CHRS informs user that the recruitment post already exists.
Use case resumes at step 1.
-
-
1b. CHRS detects invalid input format or missing details.
-
1b1. CHRS shows an error message containing either the details of the command usage or parameters constraints.
Use case resumes at step 1.
-
Actor: HR Staff (Handling recruitment)
Use case: UC12 – Delete recruitment post
MSS
-
User requests to list recruitment posts
-
CHRS shows a list of recruitment posts
-
User requests to delete a recruitment post
-
CHRS deletes all details of the recruitment post
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. CHRS shows an error message.
Use case resumes at step 2.
-
Actor: HR Staff (Handling recruitment)
Use case: UC13 - Edit recruitment post
MSS
-
User requests to list recruitment posts
-
CHRS shows a list of recruitment posts
-
User requests to edit a specific recruitment post in the list
-
CHRS updates relevant data of the recruitment post
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. CHRS shows an error message.
Use case resumes at step 2.
-
-
3b. CHRS detects invalid input format or missing details.
-
3b1. CHRS shows an error message containing either the details of the command usage or parameters constraints.
Use case resumes at step 2.
-
Use case: UC14 - Add schedules
MSS
-
User requests to add schedule for a certain employee
-
CHRS stores the schedule for that employee
Use case ends.
Extensions
-
1a. CHRS detects that requested employee does not exist.
-
1a1. CHRS informs user that the employee does not exist.
Use case resumes at step 1.
-
-
1b. CHRS detects invalid input format or missing details.
-
1b1. CHRS shows an error message containing either the details of the command usage or parameters constraints.
Use case resumes at step 1.
-
-
1c. CHRS detects that the schedule already exists.
-
1c1. CHRS informs user that the schedule already exists.
Use case resumes at step 1.
-
Use case: UC15 - Delete schedules
MSS
-
User requests to list schedules
-
CHRS shows a list of schedules
-
User requests to delete schedule of a certain employee
-
CHRS deletes the schedule of that employee in the system
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. CHRS shows an error message.
Use case resumes at step 2.
-
Use case; UC16 - Calculate leaves taken by employee
MSS
-
User requests to calculate the amount of leaves taken by a specific employee in a certain year
-
CHRS shows the amount of leaves taken by that employee within that year
Use case ends.
Extensions
-
1a. CHRS detects that requested employee does not exist.
-
1a1. CHRS informs user that the employee does not exist.
Use case resumes at step 1.
-
Appendix E: Non Functional Requirements
-
Should have different user access rights
-
Should be able to run on both 32-bit and 64-bit OS
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should be able to hold up to 1000 persons and still have a response time of 2 seconds for typical usage.
-
A user with above average typing speed, for instance 60 words per minute, for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
Appendix G: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
G.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
G.2. Adding an employee
-
Adding an employee while the storage is empty
-
Prerequisites: There must be no existing data in the system and the following
add
command must be executed first prior to all test cases.-
clear
-
add id/999999 n/Amanda Chan dob/16/06/1995 d/Finance r/Intern p/92346666 a/21 Lower Kent Ridge Rd e/amandachan@gmail.com s/1000.00
-
-
Test case:
add id/999999 n/Amanda dob/31/07/1995 d/Finance r/Intern p/92346668 a/21 Lower Kent Ridge Rd e/amanda@gmail.com s/1000.00
Expected: No employee is added. Error details shown in the status message. The details of the employee with name "Amanda Chan" added during the prerequisite shown on Employees column. Status bar remains the same. -
Test case:
add id/999998 n/Amanda dob/31/07/1995 d/Finance r/Intern p/92346668 a/21 Lower Kent Ridge Rd e/amandachan@gmail.com s/1000.00
Expected: No employee is added. Error details shown in the status message. The details of the employee with name "Amanda Chan" added during the prerequisite shown on Employees column. Status bar remains the same. -
Test case:
add id/888888 n/Belinda Chan dob/30/06/1995 d/Finance r/Intern p/91234555 a/21 Lower Kent Ridge Rd e/belinda@gmail.com s/1000.00
Expected: Employee is added to the list. Details of the added employee shown in the status message. The details of all employees shown on Employees column. Timestamp in the status bar is updated.
-
G.3. Editing an employee
-
Editing an employee while the storage is empty
-
Prerequisites: There must be no existing data in the system and the following commands must be executed first prior to all test cases.
-
clear
-
add id/999999 n/Amanda Chan dob/16/06/1995 d/Finance r/Intern p/92346666 a/21 Lower Kent Ridge Rd e/amandachan@gmail.com s/1000.00
-
add id/888888 n/Belinda Chan dob/30/06/1995 d/Finance r/Intern p/91234555 a/21 Lower Kent Ridge Rd e/belinda@gmail.com s/1000.00
-
list
-
-
Test case:
edit 1 d/Human Resource r/Manager
Expected: First employee’s details is updated on the list. Details of the edited employee shown in the status message. The details of all employees shown on Employees column. Timestamp in the status bar is updated. -
Test case:
list
followed byedit 1 e/belinda@gmail.com
Expected: No employee is edited. Error details shown in the status message. The details of the employee with name "Belinda Chan" added during the prerequisite shown on Employees column. Status bar remains the same. -
Test case:
edit 0
Expected: No changes on Employees column. No employee is edited. Error details shown in status message. Status bar remains the same.
-
G.4. Filtering employees of a certain department and position
-
Filtering employees while storage is empty
-
Prerequisites: There must be no existing data in the system and the following commands must be executed first prior to all test cases.
-
clear
-
add id/999999 n/Amanda Chan dob/16/06/1995 d/Finance r/Intern p/92346666 a/21 Lower Kent Ridge Rd e/amandachan@gmail.com s/1000.00
-
add id/888888 n/Belinda Chan dob/30/06/1995 d/Finance r/Manager p/91234555 a/21 Lower Kent Ridge Rd e/belinda@gmail.com s/1000.00
-
list
-
-
Test case:
filter asc d/finance r/intern
Expected: The details of the employee with name "Amanda Chan" added during the prerequisite shown on Employees column. Number of employees listed shown in the status message. Status bar remains the same. -
Test case:
filter asc d/Human Resource
Expected: No employees will be listed under Employees column. Number of employees listed and available departments in CHRS shown in the status message. Status bar remains the same. -
Test case:
filter dsc d/finance d/human
Expected: No changes on Employees column. Error details shown in the status message. Status bar remains the same.
-
G.5. Finding a specific employee
-
Filtering employees while storage is empty
-
Prerequisites: There must be no existing data in the system and the following commands must be executed first prior to all test cases.
-
clear
-
add id/999999 n/Amanda Chan dob/16/06/1995 d/Finance r/Intern p/92346666 a/21 Lower Kent Ridge Rd e/amandachan@gmail.com s/1000.00
-
add id/888888 n/Belinda Chan dob/30/06/1995 d/Finance r/Manager p/91234555 a/21 Lower Kent Ridge Rd e/belinda@gmail.com s/1000.00
-
list
-
-
Test case:
find amanda chan
Expected: The details of the employee with name "Amanda Chan" added during the prerequisite shown in Employees column. Number of employees listed shown in the status message. Status bar remains the same. -
Test case:
find 888888
Expected: The details of the employee with name "Belinda Chan" added during the prerequisite shown in Employees column. Number of employees listed shown in the status message. Status bar remains the same. -
Test case:
find 12345678
Expected: No changes on Employees column. Error details shown in the status message. Status bar remains the same.
-
G.6. Selecting an employee
-
Selecting an employee while all employees are listed
-
Prerequisites: Ensure the storage is not empty and list all employees using the
list
command. Multiple employees should be in the list. -
Test case: selectPerson 1
Expected: First employee is selected from the list. Selected employee index shown in the status message. Status bar remains the same. -
Test case: selectPerson 0
Expected: If there are no employees selected previously, no employees will be selected. Else, the previously selected employee will remain selected. Error details shown in the status message. Status bar remains the same. -
Other incorrect
selectPerson
commands to try:selectPerson
,selectPerson x
(where x is larger than the list size)
Expected: Similar to previous.
-
G.7. Modifying an employee’s pay
-
Modifying an employee’s pay
-
Prerequisites: There must be at least 1 employee in the list. Salary are not allowed to exceed 999999.99(max value) or goes below 0.01(min value).
Allocated Bonus should not be more than 24 months of Salary. -
Test case: modifyPay 1 s/1000
Expected: First employee’s salary will be increased by 1000 if max salary value are not exceeded after modification. Timestamp in the status bar is updated. Else, error details shown in the status message. Status bar remains the same. -
Test case: modifyPay 1 s/-1000
Expected: First employee’s salary will be decreased by 1000 if salary does not goes below min value after modification. Timestamp in the status bar is updated. Else, error details shown in the status message. Status bar remains the same. -
Test case: modifyPay 1 s/%100
Expected: First employee’s salary will be doubled if the salary does not exceed the max value after modification. Timestamp in the status bar is updated. Else, error details shown in the status message. Status bar remains the same. -
Test case: modifyPay 1 s/%-5
Expected: First employee’s salary will be decreased by 5%. Timestamp in the status bar is updated. -
Test case: modifyPay 1 b/2
Expected: First employee’s bonus will be changed to 2 months of current bonus. Timestamp in the status bar is updated. -
Test case: modifyPay 1 b/25
Expected: Error details shown in the status message. Status bar remains the same. -
Test case: modifyPay 1 s/1000 b/2
Expected: If max salary value are not exceeded after modification, first employee’s salary increased by 1000 and bonus value changed to 2 months of new salary. Timestamp in the status bar is updated. Else, error details shown in the status message. Status bar remains the same.
-
G.8. Modifying the pay of all the employees in the list
-
Modifying an employee’s pay
-
Prerequisites: There must be at least 1 employee in the list. Salary are not allowed to exceed 999999.99(max value) or goes below 0.01(min value).
Allocated Bonus should not be more than 24 months of Salary. -
Test case: modifyAllPay s/1000
Expected: All the employees' salary shown in the list will be increased by 1000 if none of the employee salary in the list exceed max salary value after modification. Timestamp in the status bar is updated. Else, error details shown in the status message. Status bar remains the same. -
Test case: modifyAllPay s/-1000
Expected: All the employees' salary shown in the list will be decreased by 1000 if none of the employee salary goes below min value after modification. Timestamp in the status bar is updated. Else, error details shown in the status message. Status bar remains the same. -
Test case: modifyAllPay s/%100
Expected: All the employees' salary shown in the list will be doubled if none of the employee salary exceed the max value after modification. Timestamp in the status bar is updated. Else, error details shown in the status message. Status bar remains the same. -
Test case: modifyAllPay s/%-5
Expected: All the employees' salary shown in the list will be decreased by 5%. Timestamp in the status bar is updated. -
Test case: modifyAllPay b/2
Expected: All the employees' bonus shown in the list will be changed to 2 months of current bonus. Timestamp in the status bar is updated. -
Test case: modifyAllPay b/25
Expected: Error details shown in the status message. Status bar remains the same. -
Test case: modifyAllPay s/1000 b/2
Expected: If max salary value are not exceeded for any employee shown in the list, All the employees' salary shown in the list will be increased by 1000 and bonus value changed to 2 months of new salary. Timestamp in the status bar is updated. Else, error details shown in the status message. Status bar remains the same.
-
G.9. Add Schedule
-
Add a single leave schedule
-
Prerequisites: There must be at least 3 valid employees in the observable employee list panel, employee id 000001 among one of the employees and a empty schedule list.
-
Test case: addSchedule id/000001 d/02/02/2019 t/LEAVE
Expected: New schedule added: Employee Id:000001 Date: 02/02/2019 Type: LEAVE -
Test case: addSchedule id/000001 d/04/02/2019 t/WORK
Expected: New schedule added: Employee Id:000001 Date: 04/02/2019 Type: WORK
-
G.10. Add Works
-
Add multiple work schedules for multiple employees.
-
Prerequisites: Completed
addSchedule
manual test above.-
Test case: addWorks d/02/02/2019 d/03/03/2019
Expected: New working schedules added for SOME of the observable employees whom are not yet added date: [02/02/2019, 03/03/2019] Unable to schedule work for the following employees below whom are on leave: Employee Id: 000001 Has leave on: [02/02/2019]
-
-
G.11. Add Leaves
-
Add multiple leave schedules for multiple employees.
-
Prerequisites: Completed
addWorks
manual test above. -
Test case: addLeaves d/04/02/2019 d/05/02/2019
Expected: New leave schedules added for SOME of the observable employees whom are not yet added date: [04/02/2019, 05/02/2019] Unable to schedule work for the following employees below whom are on work: Employee Id: 000001 Has work on: [04/02/2019]
-
G.12. Calculate Leaves
-
Calculate leaves taken by an employee in a year.
-
Prerequisites: Completed
addLeaves
manual test above. -
Test case: calculateLeaves id/000001 y/2019
Expected: Number of leaves scheduled for Employee 000001 year 2019 is: 2.
-
G.13. Select Schedule
-
Select a schedule
-
Prerequisites: Completed
calculateLeaves
manual test above. -
Test case: selectSchedule 1
Expected: Selected Schedule: 1
-
G.14. Delete Schedule
-
Delete a schedule
-
Prerequisites: Completed
selectSchedule
manual test above.-
Test Case: deleteSchedule 1
Expected: Deleted Schedule: Date: 02/02/2019 Type: LEAVE
-
-
G.15. Delete Works
-
Delete multiple work schedules
-
Prerequisites: Completed
deleteSchedule
manual test above. -
Test Case: deleteWorks d/02/02/2019 d/03/03/2019
Expected: Working schedule deleted for all observable employees that contain date : [02/02/2019, 03/03/2019]
-
G.16. Delete Leaves
-
Delete multiple leave schedules
-
Prerequisites: Completed
deleteWorks
manual test above. -
Test Case: deleteLeaves d/04/02/2019 d/05/02/2019
Expected: Leaves deleted for all observable employees that contain date : [04/02/2019, 05/02/2019]
-
G.17. Add Expenses
-
Add an expenses or modify existing expenses
-
Prerequisites: Employee with employeeId "000001" must exist in person list and doesn’t have an expenses in the expenses list
-
Test Case: addExpenses id/000001 tra/50 med/100 misc/150
Expected: Travel Expenses has value of 50.00. Medical Expenses has value of 100.00. Miscellaneous Expenses has value of 150.00. Total Expenses has value of 300.00. Timestamp in the status bar is updated.Test Case: addExpenses id/000001 tra/100 med/50 misc/25
Expected: Travel expenses is increased to 150.00, medical expenses is increased to 150.00 and miscellaneous expenses is increased to 175.00, total expenses is increased to 475.00. Timestamp in the status bar is updated.
-
G.18. Delete Expenses
-
Delete an expenses
-
There must be at least 1 employee in the observable expenses list panel
-
Test Case: deleteExpenses 1
Expected: Deletes expenses claim from expenses list with Index '1' in the expenses list.
-
G.19. Select Expenses
-
Select an expenses
-
There must be at least 1 employee in the observable expenses list panel
-
Test Case: selectExpenses 1
Expected: Select an expenses claim from expenses list with Index '1' in the expenses list.
-
G.20. Add A Recruitment Post
-
Add a single recruitment post
-
Prerequisites: none of the fields should be blank. As for job position field, it takes in only characters with maximum 20 character limits. As for working experience field, it takes in only integers from 0 to 30. As for job description field, it takes in only characters with character limits from 1 to 200 and punctuation marks including only comma, full stop and single right quote. At least one field should be different if there are more than 1 post added.
-
Test case: addRecruitmentPost jp/IT Manager me/5 jd/To maintain the network server, and company’s IT framework.
Expected: New recruitment post is added: Job Position: IT Manager Minimal years of working experience: 5 Job Description: To maintain the network server, and company’s IT framework. -
Test case: addRecruitmentPost jp/IT Manager1 me/5 jd/To maintain the network server in company
Expected: Job Position accepts only characters. It should not include numbers or should not be blank. And the maximum length of the job position is 20 characters -
Test case: addRecruitmentPost jp/IT Manager me/31 jd/To maintain the network server in company
Expected: Working Experience should only contain integers with length of at least 1 digit longAnd the range of the working experience is from 0 to 30 -
Test case: addRecruitmentPost jp/IT Manager me/-1 jd/To maintain the network server in company
Expected: Working Experience should only contain integers with length of at least 1 digit longAnd the range of the working experience is from 0 to 30 -
Test case: addRecruitmentPost jp/IT Manager me/5 jd/To maintain the network server in company
Expected: Working Experience should only contain integers with length of at least 1 digit longAnd the range of the working experience is from 0 to 30 -
Test case: addRecruitmentPost jp/IT Manager me/5 jd/To maintain the network server in company!
Expected: Job description accepts only characters. It should not include numbers or should not be blank. For the purpose of using punctuation marks, it only allows comma, full stop and single right quote. The length of job description is from 1 to 200 characters.
-
G.21. Edit A Recruitment Post
-
Edit a single recruitment post
-
Prerequisites: at least one recruitment post has been added to the recruitmentPost list panel. Minimal one field should be different from the existing recruitment post that is about to be edited.
-
Test case: editRecruitmentPost 1 jp/Accountants me/5 jd/To maintain the network server, and company’s IT framework.
Expected: Edited Recruitment Post: Job Position: Accountants Minimal years of working experience: 5 Job Description: To maintain the network server, and company’s IT framework. -
Test case: editRecruitmentPost 1 jp/Accountants me/5 jd/To maintain the network server, and company’s IT framework.
Expected: This job position already exists in the address book -
Test case: editRecruitmentPost 0 jp/Accountants me/5 jd/To maintain the network server, and company’s IT framework.
Expected: Invalid command format! editRecruitmentPost: Edits the details of the recruitment post identified by the index number used in the displayed recruitment list. Existing values will be overwritten by the input values. Parameters: INDEX (must be a positive integer) [jp/Job Position] [me/Minimal Working experience] [jd/Job description] Example: editRecruitmentPost 1 jp/IT Manager me/3 jd/To maintain the company server
-
G.22. Select A Recruitment Post
-
Select a recruitment post
-
Prerequisites: at least one recruitment post should be added before performing selectRecruitPostCommand.
-
Test case: selectRecruitmentPost 1
Expected: Selected Recruitment Post: 1
-
G.23. Delete A Recruitment Post
-
Delete a recruitment post
-
Prerequisites: at least one recruitment post should be added before performing deleteRecruitmentPostCommand.
-
Test Case: deleteRecruitmentPost 1
Expected: Deleted Recruitment Post: Job Position: Accountants Minimal years of working experience: 5 Job Description: To maintain the network server, and company’s IT framework.
-
-
G.24. Deleting an employee
-
Deleting an employee while all employees are listed
-
Prerequisites: List all employees using the
list
command. Multiple employees in the list. -
Test case:
delete 1
Expected: First employee is deleted from the list. Details of the deleted contact shown in the status message. Expenses and schedule related to the employee are also deleted from the ExpensesList and ScheduleList respectively. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No employee is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size)
Expected: Similar to previous.
-